#include <BlockingFile.h>
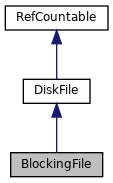
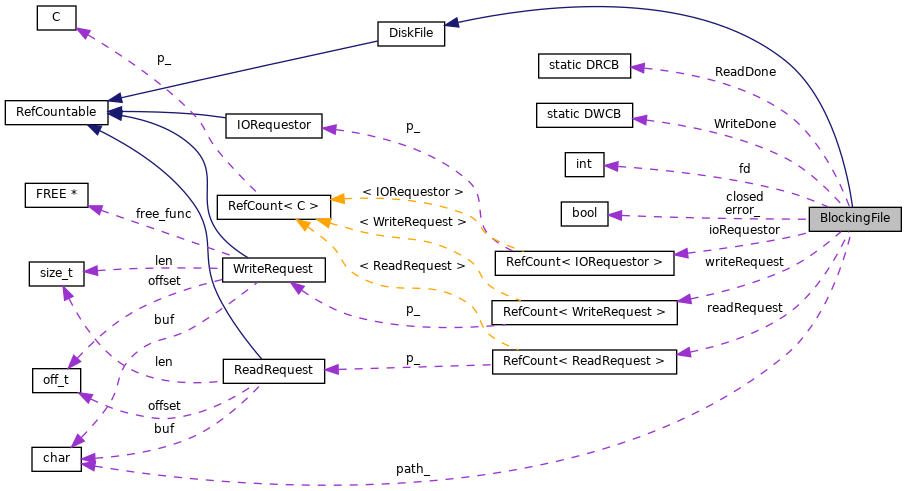
Public Types | |
typedef RefCount< DiskFile > | Pointer |
Public Member Functions | |
BlockingFile (char const *path) | |
~BlockingFile () override | |
void | open (int flags, mode_t mode, RefCount< IORequestor > callback) override |
void | create (int flags, mode_t mode, RefCount< IORequestor > callback) override |
void | read (ReadRequest *) override |
void | write (WriteRequest *) override |
void | close () override |
bool | error () const override |
int | getFD () const override |
bool | canRead () const override |
bool | ioInProgress () const override |
virtual void | configure (const Config &) |
notes supported configuration options; kids must call this first More... | |
virtual bool | canWrite () const |
Private Member Functions | |
CBDATA_CLASS (BlockingFile) | |
void | error (bool const &) |
void | doClose () |
void | readDone (int fd, const char *buf, int len, int errflag) |
void | writeDone (int fd, int errflag, size_t len) |
Private Attributes | |
int | fd |
bool | closed |
bool | error_ |
const char * | path_ |
RefCount< IORequestor > | ioRequestor |
RefCount< ReadRequest > | readRequest |
RefCount< WriteRequest > | writeRequest |
Static Private Attributes | |
static DRCB | ReadDone |
static DWCB | WriteDone |
Detailed Description
Definition at line 19 of file BlockingFile.h.
Member Typedef Documentation
◆ Pointer
|
inherited |
Definition at line 39 of file DiskFile.h.
Constructor & Destructor Documentation
◆ BlockingFile()
BlockingFile::BlockingFile | ( | char const * | path | ) |
◆ ~BlockingFile()
|
override |
Definition at line 32 of file BlockingFile.cc.
Member Function Documentation
◆ canRead()
|
overridevirtual |
◆ canWrite()
|
inlinevirtualinherited |
Reimplemented in IpcIoFile, DiskThreadsDiskFile, and MmappedFile.
Definition at line 50 of file DiskFile.h.
◆ CBDATA_CLASS()
|
private |
◆ close()
|
overridevirtual |
Implements DiskFile.
Definition at line 79 of file BlockingFile.cc.
References assert, IORequestor::closeCompleted(), debugs, doClose(), RefCount< C >::getRaw(), and ioRequestor.
◆ configure()
|
inlinevirtualinherited |
Reimplemented in IpcIoFile.
Definition at line 42 of file DiskFile.h.
Referenced by IpcIoFile::configure().
◆ create()
|
overridevirtual |
Alias for BlockingFile::open(...)
Implements DiskFile.
Definition at line 62 of file BlockingFile.cc.
References open().
◆ doClose()
|
private |
Definition at line 68 of file BlockingFile.cc.
References closed, fd, file_close(), and store_open_disk_fd.
Referenced by close(), writeDone(), and ~BlockingFile().
◆ error() [1/2]
|
overridevirtual |
◆ error() [2/2]
|
private |
Definition at line 102 of file BlockingFile.cc.
References error_.
◆ getFD()
|
inlineoverridevirtual |
During migration only
Reimplemented from DiskFile.
Definition at line 32 of file BlockingFile.h.
References fd.
◆ ioInProgress()
|
overridevirtual |
Inform callers if there is IO in progress
- Return values
-
false IO is never pending with UFS
Implements DiskFile.
Definition at line 140 of file BlockingFile.cc.
◆ open()
|
overridevirtual |
Implements DiskFile.
Definition at line 39 of file BlockingFile.cc.
References closed, debugs, error(), fd, file_open(), IORequestor::ioCompletedNotification(), ioRequestor, path_, and store_open_disk_fd.
Referenced by create().
◆ read()
|
overridevirtual |
Implements DiskFile.
Definition at line 108 of file BlockingFile.cc.
References assert, ReadRequest::buf, debugs, fd, file_read(), RefCount< C >::getRaw(), ioRequestor, ReadRequest::len, ReadRequest::offset, ReadDone, and readRequest.
◆ readDone()
Definition at line 149 of file BlockingFile.cc.
References assert, debugs, DISK_EOF, DISK_OK, fd, ioRequestor, IORequestor::readCompleted(), and readRequest.
◆ write()
|
overridevirtual |
Implements DiskFile.
Definition at line 126 of file BlockingFile.cc.
References WriteRequest::buf, debugs, fd, file_write(), WriteRequest::free_func, WriteRequest::len, WriteRequest::offset, WriteDone, and writeRequest.
◆ writeDone()
Definition at line 181 of file BlockingFile.cc.
References assert, DBG_CRITICAL, debugs, DISK_ERROR, DISK_OK, doClose(), fd, ioRequestor, IORequestor::writeCompleted(), and writeRequest.
Member Data Documentation
◆ closed
|
private |
Definition at line 41 of file BlockingFile.h.
◆ error_
|
private |
Definition at line 43 of file BlockingFile.h.
Referenced by error().
◆ fd
|
private |
Definition at line 40 of file BlockingFile.h.
Referenced by canRead(), doClose(), error(), getFD(), open(), read(), readDone(), write(), and writeDone().
◆ ioRequestor
|
private |
Definition at line 45 of file BlockingFile.h.
Referenced by close(), open(), read(), readDone(), and writeDone().
◆ path_
|
private |
Definition at line 44 of file BlockingFile.h.
Referenced by BlockingFile(), open(), and ~BlockingFile().
◆ ReadDone
|
staticprivate |
Definition at line 38 of file BlockingFile.h.
Referenced by read().
◆ readRequest
|
private |
Definition at line 46 of file BlockingFile.h.
Referenced by read(), and readDone().
◆ WriteDone
|
staticprivate |
Definition at line 39 of file BlockingFile.h.
Referenced by write().
◆ writeRequest
|
private |
Definition at line 47 of file BlockingFile.h.
Referenced by write(), and writeDone().
The documentation for this class was generated from the following files:
- src/DiskIO/Blocking/BlockingFile.h
- src/DiskIO/Blocking/BlockingFile.cc
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products