A map of MemMapSlots indexed by their keys, with read/write slot locking. More...
#include <MemMap.h>
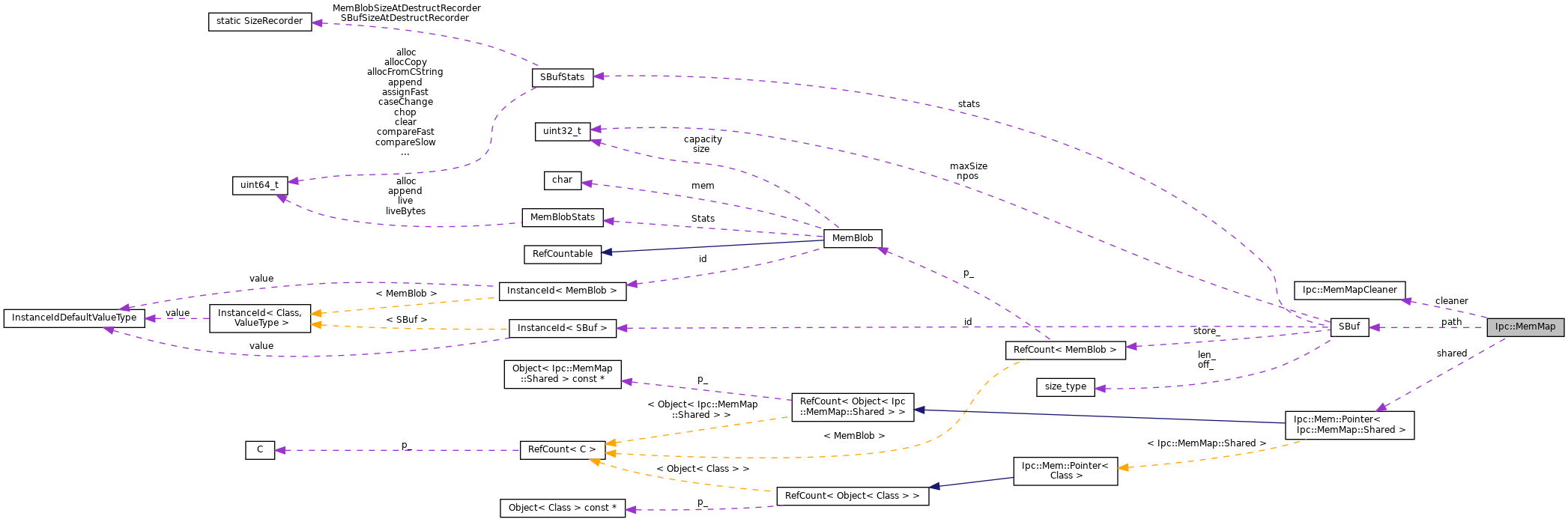
Classes | |
class | Shared |
data shared across maps in different processes More... | |
Public Types | |
typedef MemMapSlot | Slot |
typedef Mem::Owner< Shared > | Owner |
Public Member Functions | |
MemMap (const char *const aPath) | |
Slot * | openForWriting (const cache_key *const key, sfileno &fileno) |
Slot * | openForWritingAt (sfileno fileno, bool overwriteExisting=true) |
void | closeForWriting (const sfileno fileno) |
successfully finish writing the entry More... | |
void | switchWritingToReading (const sfileno fileno) |
stop writing the locked entry and start reading it More... | |
const Slot * | peekAtReader (const sfileno fileno) const |
only works on locked entries; returns nil unless the slot is readable More... | |
void | free (const sfileno fileno) |
mark the slot as waiting to be freed and, if possible, free it More... | |
const Slot * | openForReading (const cache_key *const key, sfileno &fileno) |
open slot for reading, increments read level More... | |
const Slot * | openForReadingAt (const sfileno fileno) |
open slot for reading, increments read level More... | |
void | closeForReading (const sfileno fileno) |
close slot after reading, decrements read level More... | |
bool | full () const |
there are no empty slots left More... | |
bool | valid (const int n) const |
whether n is a valid slot coordinate More... | |
int | entryCount () const |
number of used slots More... | |
int | entryLimit () const |
maximum number of slots that can be used More... | |
void | updateStats (ReadWriteLockStats &stats) const |
adds approximate current stats to the supplied ones More... | |
Static Public Member Functions | |
static Owner * | Init (const char *const path, const int limit) |
initialize shared memory More... | |
Public Attributes | |
MemMapCleaner * | cleaner |
Static Protected Member Functions | |
static Owner * | Init (const char *const path, const int limit, const size_t extrasSize) |
Protected Attributes | |
const SBuf | path |
cache_dir path, used for logging More... | |
Mem::Pointer< Shared > | shared |
Private Member Functions | |
int | slotIndexByKey (const cache_key *const key) const |
Slot & | slotByKey (const cache_key *const key) |
Slot * | openForReading (Slot &s) |
void | abortWriting (const sfileno fileno) |
terminate writing the entry, freeing its slot for others to use More... | |
void | freeIfNeeded (Slot &s) |
void | freeLocked (Slot &s, bool keepLocked) |
unconditionally frees the already exclusively locked slot and releases lock More... | |
Detailed Description
Member Typedef Documentation
◆ Owner
typedef Mem::Owner<Shared> Ipc::MemMap::Owner |
◆ Slot
typedef MemMapSlot Ipc::MemMap::Slot |
Constructor & Destructor Documentation
◆ MemMap()
Ipc::MemMap::MemMap | ( | const char *const | aPath | ) |
Member Function Documentation
◆ abortWriting()
|
private |
Definition at line 114 of file MemMap.cc.
References assert, debugs, and Ipc::MemMapSlot::writing().
◆ closeForReading()
void Ipc::MemMap::closeForReading | ( | const sfileno | fileno | ) |
Definition at line 207 of file MemMap.cc.
References assert, debugs, Ipc::MemMapSlot::lock, Ipc::MemMapSlot::reading(), and Ipc::ReadWriteLock::unlockShared().
Referenced by get_session_cb(), and remove_session_cb().
◆ closeForWriting()
void Ipc::MemMap::closeForWriting | ( | const sfileno | fileno | ) |
Definition at line 91 of file MemMap.cc.
References assert, debugs, Ipc::MemMapSlot::lock, Ipc::ReadWriteLock::unlockExclusive(), and Ipc::MemMapSlot::writing().
Referenced by store_session_cb().
◆ entryCount()
◆ entryLimit()
◆ free()
void Ipc::MemMap::free | ( | const sfileno | fileno | ) |
Definition at line 138 of file MemMap.cc.
References assert, debugs, Ipc::MemMapSlot::lock, Ipc::ReadWriteLock::lockExclusive(), and Ipc::MemMapSlot::waitingToBeFreed.
Referenced by remove_session_cb().
◆ freeIfNeeded()
|
private |
◆ freeLocked()
|
private |
Definition at line 276 of file MemMap.cc.
References debugs, Ipc::MemMapSlot::empty(), Ipc::MemMapSlot::key, Ipc::MemMapSlot::lock, Ipc::ReadWriteLock::unlockExclusive(), and Ipc::MemMapSlot::waitingToBeFreed.
◆ full()
◆ Init() [1/2]
|
static |
Definition at line 36 of file MemMap.cc.
References Acl::Init().
Referenced by SharedSessionCacheRr::create().
◆ Init() [2/2]
|
staticprotected |
◆ openForReading() [1/2]
const Ipc::MemMap::Slot * Ipc::MemMap::openForReading | ( | const cache_key *const | key, |
sfileno & | fileno | ||
) |
Definition at line 153 of file MemMap.cc.
References debugs, and storeKeyText().
Referenced by get_session_cb(), and remove_session_cb().
◆ openForReading() [2/2]
◆ openForReadingAt()
const Ipc::MemMap::Slot * Ipc::MemMap::openForReadingAt | ( | const sfileno | fileno | ) |
Definition at line 174 of file MemMap.cc.
References assert, debugs, Ipc::MemMapSlot::empty(), Ipc::MemMapSlot::lock, Ipc::ReadWriteLock::lockShared(), Ipc::ReadWriteLock::unlockShared(), and Ipc::MemMapSlot::waitingToBeFreed.
◆ openForWriting()
Ipc::MemMap::Slot * Ipc::MemMap::openForWriting | ( | const cache_key *const | key, |
sfileno & | fileno | ||
) |
finds, locks and return a slot for an empty key position, erasing the old entry (if any)
Definition at line 42 of file MemMap.cc.
References debugs, and storeKeyText().
Referenced by store_session_cb().
◆ openForWritingAt()
Ipc::MemMap::Slot * Ipc::MemMap::openForWritingAt | ( | sfileno | fileno, |
bool | overwriteExisting = true |
||
) |
locks and returns a slot for the empty fileno position; if overwriteExisting is false and the position is not empty, returns nil
Definition at line 57 of file MemMap.cc.
References assert, debugs, Ipc::MemMapSlot::empty(), Ipc::MemMapSlot::lock, Ipc::ReadWriteLock::lockExclusive(), Ipc::MemMapSlot::reading(), Ipc::ReadWriteLock::unlockExclusive(), Ipc::MemMapSlot::waitingToBeFreed, and Ipc::MemMapSlot::writing().
◆ peekAtReader()
const Ipc::MemMap::Slot * Ipc::MemMap::peekAtReader | ( | const sfileno | fileno | ) | const |
Definition at line 125 of file MemMap.cc.
References assert, Ipc::MemMapSlot::reading(), and Ipc::MemMapSlot::writing().
◆ slotByKey()
|
private |
◆ slotIndexByKey()
Definition at line 262 of file MemMap.cc.
References hash_key(), and MEMMAP_SLOT_KEY_SIZE.
◆ switchWritingToReading()
void Ipc::MemMap::switchWritingToReading | ( | const sfileno | fileno | ) |
Definition at line 102 of file MemMap.cc.
References assert, debugs, Ipc::MemMapSlot::lock, Ipc::ReadWriteLock::switchExclusiveToShared(), and Ipc::MemMapSlot::writing().
◆ updateStats()
void Ipc::MemMap::updateStats | ( | ReadWriteLockStats & | stats | ) | const |
Definition at line 236 of file MemMap.cc.
References Ping::stats.
◆ valid()
Member Data Documentation
◆ cleaner
MemMapCleaner* Ipc::MemMap::cleaner |
The cleaner MemMapCleaner::noteFreeMapSlot method called when a readable entry is freed.
◆ path
|
protected |
◆ shared
|
protected |
The documentation for this class was generated from the following files: