#include <StoreMap.h>
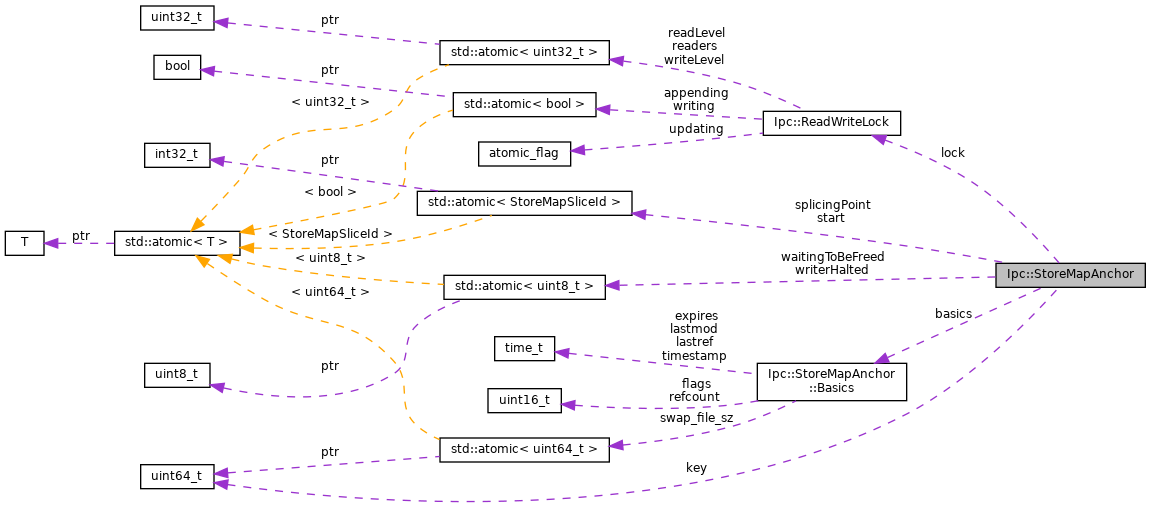
Classes | |
struct | Basics |
Public Member Functions | |
StoreMapAnchor () | |
void | set (const StoreEntry &anEntry, const cache_key *aKey=nullptr) |
store StoreEntry key and basics for an inode slot More... | |
void | exportInto (StoreEntry &) const |
load StoreEntry basics that were previously stored with set() More... | |
void | setKey (const cache_key *const aKey) |
bool | sameKey (const cache_key *const aKey) const |
void | rewind () |
undo the effects of set(), setKey(), etc., but keep locks and state More... | |
bool | empty () const |
bool | reading () const |
bool | writing () const |
bool | complete () const |
Public Attributes | |
ReadWriteLock | lock |
protects slot data below More... | |
std::atomic< uint8_t > | waitingToBeFreed |
std::atomic< uint8_t > | writerHalted |
whether StoreMap::abortWriting() was called for a read-locked entry More... | |
uint64_t | key [2] = {0, 0} |
StoreEntry key. More... | |
struct Ipc::StoreMapAnchor::Basics | basics |
std::atomic< StoreMapSliceId > | start |
where the chain of StoreEntry slices begins [app] More... | |
std::atomic< StoreMapSliceId > | splicingPoint |
Detailed Description
Maintains shareable information about a StoreEntry as a whole. An anchor points to one or more StoreEntry slices. This is the only lockable part of shared StoreEntry information, providing protection for all StoreEntry slices.
Definition at line 56 of file StoreMap.h.
Constructor & Destructor Documentation
◆ StoreMapAnchor()
Ipc::StoreMapAnchor::StoreMapAnchor | ( | ) |
Definition at line 938 of file StoreMap.cc.
Member Function Documentation
◆ complete()
|
inline |
Definition at line 77 of file StoreMap.h.
References empty(), and writing().
Referenced by Rock::SwapDir::anchorEntry(), MemStore::anchorEntry(), MemStore::copyFromShm(), and Ipc::StoreMap::switchWritingToReading().
◆ empty()
|
inline |
Definition at line 74 of file StoreMap.h.
References key.
Referenced by Ipc::StoreMap::compareVersions(), complete(), Ipc::StoreMap::freeChain(), Ipc::StoreMap::freeEntry(), Ipc::StoreMap::openForReadingAt(), Ipc::StoreMap::openForWritingAt(), and Ipc::StoreMap::purgeOne().
◆ exportInto()
void Ipc::StoreMapAnchor::exportInto | ( | StoreEntry & | into | ) | const |
Definition at line 978 of file StoreMap.cc.
References assert, EBIT_CLR, EBIT_SET, ENTRY_REQUIRES_COLLAPSING, StoreEntry::expires, StoreEntry::flags, StoreEntry::hittingRequiresCollapsing(), StoreEntry::lastModified(), StoreEntry::lastref, StoreEntry::refcount, StoreEntry::swap_file_sz, and StoreEntry::timestamp.
Referenced by Rock::SwapDir::anchorEntry(), and MemStore::anchorEntry().
◆ reading()
|
inline |
Definition at line 75 of file StoreMap.h.
References lock, and Ipc::ReadWriteLock::readers.
Referenced by Ipc::StoreMap::closeForReading(), Ipc::StoreMap::openForWritingAt(), Ipc::StoreMap::peekAtReader(), Ipc::StoreMap::peekAtWriter(), and Ipc::StoreMap::sliceContaining().
◆ rewind()
void Ipc::StoreMapAnchor::rewind | ( | ) |
Definition at line 1002 of file StoreMap.cc.
References assert.
Referenced by Ipc::StoreMap::forgetWritingEntry(), and Ipc::StoreMap::freeChain().
◆ sameKey()
bool Ipc::StoreMapAnchor::sameKey | ( | const cache_key *const | aKey | ) | const |
Definition at line 951 of file StoreMap.cc.
Referenced by Ipc::StoreMap::freeEntryByKey(), Ipc::StoreMap::markedForDeletion(), Ipc::StoreMap::openForReadingAt(), Rock::SwapDir::openStoreIO(), and Rock::Rebuild::sameEntry().
◆ set()
void Ipc::StoreMapAnchor::set | ( | const StoreEntry & | anEntry, |
const cache_key * | aKey = nullptr |
||
) |
Definition at line 958 of file StoreMap.cc.
References assert, EBIT_CLR, StoreEntry::expires, StoreEntry::flags, hash_link::key, KEY_PRIVATE, StoreEntry::lastModified(), StoreEntry::lastref, StoreEntry::refcount, StoreEntry::swap_file_sz, and StoreEntry::timestamp.
Referenced by Rock::SwapDir::createStoreIO(), Rock::Rebuild::importEntry(), Ipc::StoreMap::openForUpdating(), and MemStore::startCaching().
◆ setKey()
void Ipc::StoreMapAnchor::setKey | ( | const cache_key *const | aKey | ) |
Definition at line 944 of file StoreMap.cc.
References Store::Controller::markedForDeletion(), and Store::Root().
Referenced by Rock::Rebuild::primeNewEntry().
◆ writing()
|
inline |
Definition at line 76 of file StoreMap.h.
References lock, and Ipc::ReadWriteLock::writing.
Referenced by Ipc::StoreMap::abortWriting(), Ipc::StoreMap::closeForWriting(), complete(), Ipc::StoreMap::forgetWritingEntry(), Ipc::StoreMap::openForUpdating(), Ipc::StoreMap::openForWritingAt(), Ipc::StoreMap::peekAtReader(), Ipc::StoreMap::peekAtWriter(), Ipc::StoreMap::startAppending(), and Ipc::StoreMap::switchWritingToReading().
Member Data Documentation
◆ basics
struct Ipc::StoreMapAnchor::Basics Ipc::StoreMapAnchor::basics |
Referenced by Rock::Rebuild::addSlotToEntry(), Ipc::StoreMap::compareVersions(), MemStore::copyFromShm(), MemStore::copyToShmSlice(), Rock::Rebuild::finalizeOrThrow(), Rock::Rebuild::importEntry(), Rock::SwapDir::openStoreIO(), Rock::Rebuild::primeNewEntry(), MemStore::updateAnchoredWith(), and MemStore::updateHeadersOrThrow().
◆ key
uint64_t Ipc::StoreMapAnchor::key[2] = {0, 0} |
Definition at line 88 of file StoreMap.h.
Referenced by empty().
◆ lock
|
mutable |
Definition at line 80 of file StoreMap.h.
Referenced by Ipc::StoreMap::abortUpdating(), Ipc::StoreMap::abortWriting(), Ipc::StoreMap::closeForReading(), Ipc::StoreMap::closeForUpdating(), Ipc::StoreMap::closeForWriting(), Ipc::StoreMap::forgetWritingEntry(), Ipc::StoreMap::freeChain(), Ipc::StoreMap::freeEntry(), Ipc::StoreMap::freeEntryByKey(), Ipc::StoreMap::openForReadingAt(), Ipc::StoreMap::openForUpdating(), Ipc::StoreMap::openForWritingAt(), Ipc::StoreMap::purgeOne(), Transients::readers(), reading(), Ipc::StoreMap::startAppending(), Ipc::StoreMap::switchWritingToReading(), and writing().
◆ splicingPoint
std::atomic<StoreMapSliceId> Ipc::StoreMapAnchor::splicingPoint |
where the updated chain prefix containing metadata/headers ends [update] if unset, this anchor points to a chain that was never updated
Definition at line 115 of file StoreMap.h.
Referenced by Ipc::StoreMap::closeForUpdating(), Ipc::StoreMap::freeChain(), and Ipc::StoreMap::openForWritingAt().
◆ start
std::atomic<StoreMapSliceId> Ipc::StoreMapAnchor::start |
Definition at line 111 of file StoreMap.h.
Referenced by Rock::Rebuild::addSlotToEntry(), Ipc::StoreMap::closeForUpdating(), MemStore::copyFromShm(), MemStore::copyToShm(), Rock::Rebuild::finalizeOrThrow(), Rock::Rebuild::freeBadEntry(), Ipc::StoreMap::freeChain(), MemStore::nextAppendableSlice(), Ipc::StoreMap::openForWritingAt(), Rock::Rebuild::primeNewEntry(), Ipc::StoreMap::purgeOne(), and Ipc::StoreMap::sliceContaining().
◆ waitingToBeFreed
std::atomic<uint8_t> Ipc::StoreMapAnchor::waitingToBeFreed |
may be accessed w/o a lock
Definition at line 81 of file StoreMap.h.
Referenced by Ipc::StoreMap::abortWriting(), Ipc::StoreMap::closeForUpdating(), Ipc::StoreMap::freeEntry(), Ipc::StoreMap::freeEntryByKey(), Ipc::StoreMap::markedForDeletion(), Ipc::StoreMap::openForReadingAt(), and Ipc::StoreMap::openForWritingAt().
◆ writerHalted
std::atomic<uint8_t> Ipc::StoreMapAnchor::writerHalted |
Definition at line 83 of file StoreMap.h.
Referenced by Ipc::StoreMap::abortWriting(), and MemStore::copyFromShm().
The documentation for this class was generated from the following files:
- src/ipc/StoreMap.h
- src/ipc/StoreMap.cc