#include <Notes.h>
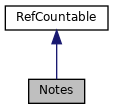
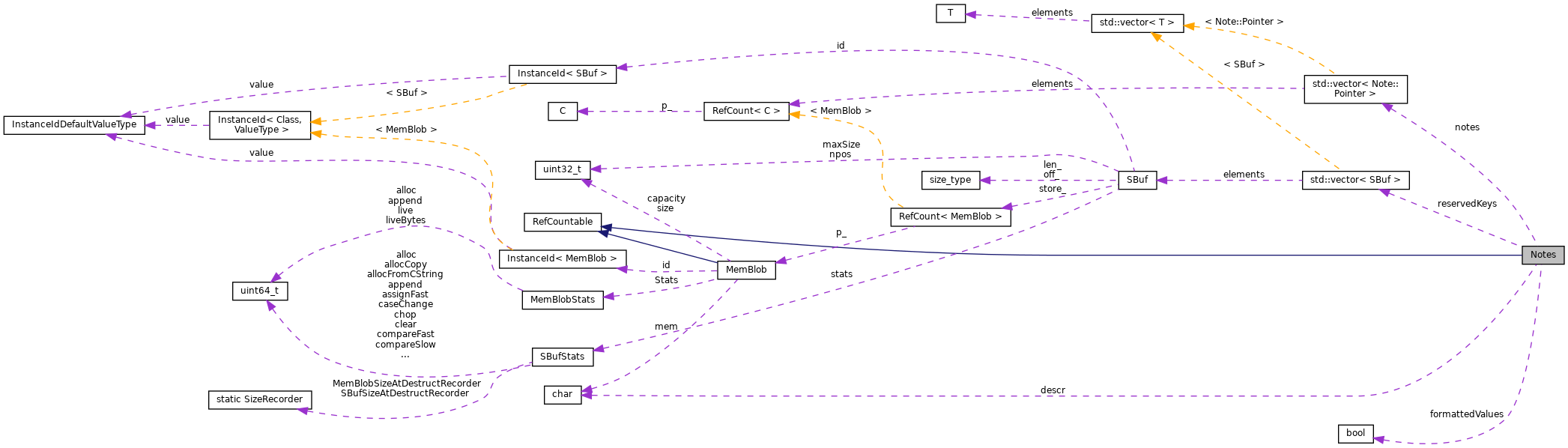
Public Types | |
typedef RefCount< Notes > | Pointer |
typedef std::vector< SBuf > | Keys |
unordered annotation names More... | |
typedef std::vector< Note::Pointer > | NotesList |
typedef NotesList::iterator | iterator |
iterates over the notes list More... | |
typedef NotesList::const_iterator | const_iterator |
iterates over the notes list More... | |
Public Member Functions | |
Notes (const char *aDescr, const Keys *extraReservedKeys=nullptr, bool allowFormatted=true) | |
Notes ()=default | |
~Notes () override | |
Notes (const Notes &)=delete | |
Notes & | operator= (const Notes &)=delete |
Note::Pointer | parse (ConfigParser &parser) |
Parses a notes line and returns a pointer to the parsed Note object. More... | |
void | parseKvPair () |
Parses an annotate line with "key=value" or "key+=value" formats. More... | |
void | dump (StoreEntry *entry, const char *name) |
Dump the notes list to the given StoreEntry object. More... | |
void | clean () |
clean the notes list More... | |
iterator | begin () |
points to the first argument More... | |
iterator | end () |
points to the end of list More... | |
bool | empty () const |
const char * | toString (const char *sep="\r\n") const |
void | updateNotePairs (NotePairsPointer pairs, const CharacterSet *delimiters, const AccessLogEntryPointer &al) |
Private Member Functions | |
void | banReservedKey (const SBuf &key, const Keys &banned) const |
Makes sure the given key is not on the given list of banned names. More... | |
void | validateKey (const SBuf &key) const |
Note::Pointer | add (const SBuf ¬eKey) |
Note::Pointer | find (const SBuf ¬eKey) |
Static Private Member Functions | |
static const Notes::Keys & | ReservedKeys () |
always prohibited key names More... | |
Private Attributes | |
NotesList | notes |
The Note::Pointer objects array list. More... | |
const char * | descr = nullptr |
identifies note source in error messages More... | |
Keys | reservedKeys |
a list of additional prohibited key names More... | |
bool | formattedValues = false |
whether to expand quoted logformat codes More... | |
Detailed Description
Member Typedef Documentation
◆ const_iterator
typedef NotesList::const_iterator Notes::const_iterator |
◆ iterator
typedef NotesList::iterator Notes::iterator |
◆ Keys
typedef std::vector<SBuf> Notes::Keys |
◆ NotesList
typedef std::vector<Note::Pointer> Notes::NotesList |
◆ Pointer
typedef RefCount<Notes> Notes::Pointer |
Constructor & Destructor Documentation
◆ Notes() [1/3]
|
explicit |
Definition at line 150 of file Notes.cc.
References reservedKeys.
◆ Notes() [2/3]
|
default |
◆ ~Notes()
◆ Notes() [3/3]
|
delete |
Member Function Documentation
◆ add()
|
private |
Adds a note to the notes list and returns a pointer to the related Note object. If the note key already exists in list, returns a pointer to the existing object. If keyLen is not provided, the noteKey is assumed null-terminated.
Definition at line 159 of file Notes.cc.
Referenced by parse(), and parseKvPair().
◆ banReservedKey()
◆ begin()
◆ clean()
|
inline |
◆ dump()
void Notes::dump | ( | StoreEntry * | entry, |
const char * | name | ||
) |
◆ empty()
|
inline |
- Returns
- true if the notes list is empty
Definition at line 139 of file Notes.h.
References notes.
Referenced by accessLogInit(), and ACLAnnotationData::empty().
◆ end()
◆ find()
|
private |
◆ operator=()
◆ parse()
Note::Pointer Notes::parse | ( | ConfigParser & | parser | ) |
Definition at line 202 of file Notes.cc.
References aclParseAclList(), add(), SBuf::append(), SBuf::c_str(), descr, ConfigParser::DisableMacros(), ConfigParser::EnableMacros(), fatalf(), formattedValues, ConfigParser::LastTokenWasQuoted(), ConfigParser::NextQuotedToken(), ConfigParser::NextToken(), and validateKey().
Referenced by parse_note().
◆ parseKvPair()
void Notes::parseKvPair | ( | ) |
Definition at line 223 of file Notes.cc.
References add(), assert, DBG_CRITICAL, debugs, descr, fatalf(), find(), formattedValues, ConfigParser::LastTokenWasQuoted(), Note::Value::mhAppend, Note::Value::mhReplace, ConfigParser::NextKvPair(), and validateKey().
Referenced by ACLAnnotationData::parse().
◆ ReservedKeys()
|
staticprivate |
◆ toString()
const char * Notes::toString | ( | const char * | sep = "\r\n" | ) | const |
Convert Notes list to a string consist of "Key: Value" entries separated by sep string.
Definition at line 262 of file Notes.cc.
References SBuf::append(), SBuf::c_str(), SBuf::clear(), SBuf::isEmpty(), and notes.
Referenced by ACLAnnotationData::dump().
◆ updateNotePairs()
void Notes::updateNotePairs | ( | NotePairsPointer | pairs, |
const CharacterSet * | delimiters, | ||
const AccessLogEntryPointer & | al | ||
) |
Definition at line 248 of file Notes.cc.
References notes.
Referenced by ACLAnnotationData::annotate().
◆ validateKey()
|
private |
Verifies that the key is not reserved (fatal error) and does not contain special characters (non-fatal error).
Definition at line 184 of file Notes.cc.
References CharacterSet::ALPHA, banReservedKey(), DBG_CRITICAL, debugs, CharacterSet::DIGIT, SBuf::findFirstNotOf(), SBuf::npos, reservedKeys, and ReservedKeys().
Referenced by parse(), and parseKvPair().
Member Data Documentation
◆ descr
|
private |
Definition at line 161 of file Notes.h.
Referenced by banReservedKey(), parse(), and parseKvPair().
◆ formattedValues
|
private |
Definition at line 164 of file Notes.h.
Referenced by parse(), and parseKvPair().
◆ notes
|
private |
◆ reservedKeys
|
private |
Definition at line 163 of file Notes.h.
Referenced by Notes(), and validateKey().
The documentation for this class was generated from the following files: