#include <RandomUuid.h>
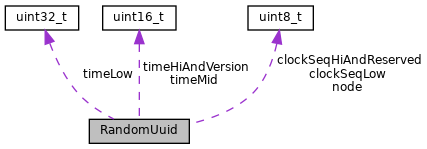
Public Types | |
using | Serialized = std::array< uint8_t, 128/8 > |
UUID representation independent of machine byte-order architecture. More... | |
Public Member Functions | |
RandomUuid () | |
creates a new unique ID (i.e. not a "nil UUID" in RFC 4122 terminology) More... | |
RandomUuid (const Serialized &) | |
imports a UUID value that was exported using the serialize() API More... | |
RandomUuid (RandomUuid &&)=default | |
RandomUuid & | operator= (RandomUuid &&)=default |
RandomUuid & | operator= (const RandomUuid &)=delete |
Serialized | serialize () const |
exports UUID value; suitable for long-term storage More... | |
bool | operator== (const RandomUuid &) const |
bool | operator!= (const RandomUuid &other) const |
RandomUuid | clone () const |
creates a UUID object with the same value as this UUID More... | |
void | print (std::ostream &os) const |
writes a human-readable representation More... | |
Private Member Functions | |
RandomUuid (const RandomUuid &)=default | |
char * | raw () |
read/write access to storage bytes More... | |
const char * | raw () const |
read-only access to storage bytes More... | |
bool | sane () const |
whether this (being constructed) object follows UUID version 4 variant 1 format More... | |
Private Attributes | |
uint32_t | timeLow |
uint16_t | timeMid |
uint16_t | timeHiAndVersion |
uint8_t | clockSeqHiAndReserved |
uint8_t | clockSeqLow |
uint8_t | node [6] |
Detailed Description
128-bit Universally Unique IDentifier (UUID), version 4 (variant 1) as defined by RFC 4122. These UUIDs are generated from pseudo-random numbers.
Definition at line 17 of file RandomUuid.h.
Member Typedef Documentation
◆ Serialized
using RandomUuid::Serialized = std::array<uint8_t, 128/8> |
Definition at line 21 of file RandomUuid.h.
Constructor & Destructor Documentation
◆ RandomUuid() [1/4]
RandomUuid::RandomUuid | ( | ) |
Definition at line 27 of file RandomUuid.cc.
References assert, clockSeqHiAndReserved, EBIT_CLR, EBIT_SET, RandomSeed64(), raw(), sane(), and timeHiAndVersion.
◆ RandomUuid() [2/4]
|
explicit |
Definition at line 55 of file RandomUuid.cc.
References Here, raw(), sane(), timeHiAndVersion, timeLow, and timeMid.
◆ RandomUuid() [3/4]
|
default |
◆ RandomUuid() [4/4]
|
privatedefault |
Member Function Documentation
◆ clone()
|
inline |
Definition at line 43 of file RandomUuid.h.
Referenced by serialize().
◆ operator!=()
|
inline |
Definition at line 40 of file RandomUuid.h.
◆ operator=() [1/2]
|
delete |
◆ operator=() [2/2]
|
default |
◆ operator==()
bool RandomUuid::operator== | ( | const RandomUuid & | other | ) | const |
Definition at line 113 of file RandomUuid.cc.
References raw().
◆ print()
void RandomUuid::print | ( | std::ostream & | os | ) | const |
Definition at line 92 of file RandomUuid.cc.
References clockSeqHiAndReserved, clockSeqLow, node, timeHiAndVersion, timeLow, and timeMid.
Referenced by operator<<().
◆ raw() [1/2]
|
inlineprivate |
Definition at line 52 of file RandomUuid.h.
Referenced by RandomUuid(), and operator==().
◆ raw() [2/2]
|
inlineprivate |
Definition at line 55 of file RandomUuid.h.
◆ sane()
|
private |
Definition at line 68 of file RandomUuid.cc.
References clockSeqHiAndReserved, EBIT_TEST, and timeHiAndVersion.
Referenced by RandomUuid(), and serialize().
◆ serialize()
RandomUuid::Serialized RandomUuid::serialize | ( | ) | const |
Definition at line 79 of file RandomUuid.cc.
References assert, clone(), sane(), timeHiAndVersion, timeLow, and timeMid.
Referenced by TestRandomUuid::testSerialization().
Member Data Documentation
◆ clockSeqHiAndReserved
|
private |
Definition at line 68 of file RandomUuid.h.
Referenced by RandomUuid(), print(), and sane().
◆ clockSeqLow
|
private |
Definition at line 69 of file RandomUuid.h.
Referenced by print().
◆ node
|
private |
Definition at line 70 of file RandomUuid.h.
Referenced by print().
◆ timeHiAndVersion
|
private |
Definition at line 67 of file RandomUuid.h.
Referenced by RandomUuid(), print(), sane(), and serialize().
◆ timeLow
|
private |
Definition at line 65 of file RandomUuid.h.
Referenced by RandomUuid(), print(), and serialize().
◆ timeMid
|
private |
Definition at line 66 of file RandomUuid.h.
Referenced by RandomUuid(), print(), and serialize().
The documentation for this class was generated from the following files:
- src/base/RandomUuid.h
- src/base/RandomUuid.cc