#include <SBuf.h>
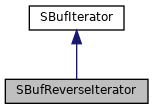
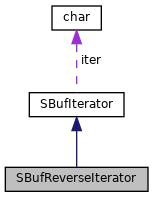
Public Types | |
using | iterator_category = std::input_iterator_tag |
using | value_type = char |
using | difference_type = std::ptrdiff_t |
using | pointer = char * |
using | reference = char & |
typedef MemBlob::size_type | size_type |
Public Member Functions | |
SBufReverseIterator & | operator++ () |
const char & | operator* () const |
bool | operator== (const SBufIterator &s) const |
bool | operator!= (const SBufIterator &s) const |
Protected Member Functions | |
SBufReverseIterator (const SBuf &s, size_type sz) | |
Protected Attributes | |
const char * | iter = nullptr |
Friends | |
class | SBuf |
Detailed Description
Reverse input const_iterator for SBufs
Please note that any operation on the underlying SBuf may invalidate all iterators over it, resulting in undefined behavior by them.
Member Typedef Documentation
◆ difference_type
|
inherited |
◆ iterator_category
|
inherited |
◆ pointer
|
inherited |
◆ reference
|
inherited |
◆ size_type
|
inherited |
◆ value_type
|
inherited |
Constructor & Destructor Documentation
◆ SBufReverseIterator()
Member Function Documentation
◆ operator!=()
|
inlineinherited |
Definition at line 788 of file SBuf.h.
References SBufIterator::iter.
◆ operator*()
|
inline |
Definition at line 82 of file SBuf.h.
References SBufIterator::iter.
◆ operator++()
|
inline |
Definition at line 81 of file SBuf.h.
References SBufIterator::iter.
◆ operator==()
|
inlineinherited |
Definition at line 781 of file SBuf.h.
References SBufIterator::iter.
Friends And Related Function Documentation
◆ SBuf
Member Data Documentation
◆ iter
|
protectedinherited |
Definition at line 69 of file SBuf.h.
Referenced by SBufIterator::operator!=(), SBufIterator::operator*(), operator*(), SBufIterator::operator++(), operator++(), and SBufIterator::operator==().
The documentation for this class was generated from the following file:
- src/sbuf/SBuf.h