#include <Stats.h>
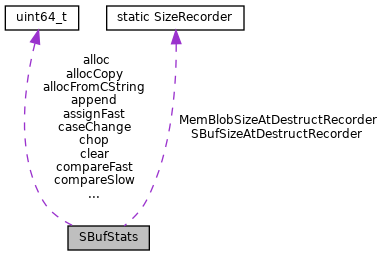
Public Types | |
using | SizeRecorder = void(*)(size_t) |
function for collecting detailed size-related statistics More... | |
Public Member Functions | |
std::ostream & | dump (std::ostream &os) const |
Dump statistics to an ostream. More... | |
SBufStats & | operator+= (const SBufStats &) |
Static Public Member Functions | |
static void | RecordSBufSizeAtDestruct (size_t) |
Record the size a SBuf had when it was destructed. More... | |
static void | RecordMemBlobSizeAtDestruct (size_t) |
Record the size a MemBlob had when it was destructed. More... | |
Public Attributes | |
uint64_t | alloc = 0 |
number of calls to SBuf constructors More... | |
uint64_t | allocCopy = 0 |
number of calls to SBuf copy-constructor More... | |
uint64_t | allocFromCString = 0 |
number of copy-allocations from c-strings More... | |
uint64_t | assignFast = 0 |
number of no-copy assignment operations More... | |
uint64_t | clear = 0 |
number of clear operations More... | |
uint64_t | append = 0 |
number of append operations More... | |
uint64_t | moves = 0 |
number of move constructions/assignments More... | |
uint64_t | toStream = 0 |
number of write operations to ostreams More... | |
uint64_t | setChar = 0 |
number of calls to setAt More... | |
uint64_t | getChar = 0 |
number of calls to at() and operator[] More... | |
uint64_t | compareSlow = 0 |
number of comparison operations requiring data scan More... | |
uint64_t | compareFast = 0 |
number of comparison operations not requiring data scan More... | |
uint64_t | copyOut = 0 |
number of data-copies to other forms of buffers More... | |
uint64_t | rawAccess = 0 |
number of accesses to raw contents More... | |
uint64_t | nulTerminate = 0 |
number of c_str() terminations More... | |
uint64_t | chop = 0 |
number of chop operations More... | |
uint64_t | trim = 0 |
number of trim operations More... | |
uint64_t | find = 0 |
number of find operations More... | |
uint64_t | caseChange = 0 |
number of toUpper and toLower operations More... | |
uint64_t | cowAvoided = 0 |
number of cow() calls requiring no expensive operations More... | |
uint64_t | cowShift = 0 |
number of cow() calls requiring just a memmove(3) inside an old buffer More... | |
uint64_t | cowJustAlloc = 0 |
number of cow() calls requiring just a new empty buffer More... | |
uint64_t | cowAllocCopy = 0 |
number of cow() calls requiring copying into a new buffer More... | |
uint64_t | live = 0 |
number of currently-allocated SBuf More... | |
Static Public Attributes | |
static SizeRecorder | SBufSizeAtDestructRecorder = nullptr |
collects statistics about SBuf sizes at SBuf destruction time More... | |
static SizeRecorder | MemBlobSizeAtDestructRecorder = nullptr |
collects statistics about MemBlob capacity at MemBlob destruction time More... | |
Detailed Description
Container for various SBuf class-wide statistics.
The stats are not completely accurate; they're mostly meant to understand whether Squid is leaking resources and whether SBuf is paying off the expected gains.
Member Typedef Documentation
◆ SizeRecorder
using SBufStats::SizeRecorder = void (*)(size_t) |
Member Function Documentation
◆ dump()
std::ostream & SBufStats::dump | ( | std::ostream & | os | ) | const |
Definition at line 65 of file Stats.cc.
References alloc, allocCopy, allocFromCString, append, assignFast, caseChange, chop, clear, compareFast, compareSlow, copyOut, cowAllocCopy, cowAvoided, cowJustAlloc, cowShift, find, getChar, MemBlob::GetStats(), MemBlobStats::live, live, moves, nulTerminate, rawAccess, setChar, toStream, and trim.
Referenced by SBufStatsAction::dump(), and TestSBuf::testDumpStats().
◆ operator+=()
Definition at line 34 of file Stats.cc.
References alloc, allocCopy, allocFromCString, append, assignFast, caseChange, chop, clear, compareFast, compareSlow, copyOut, cowAllocCopy, cowAvoided, cowJustAlloc, cowShift, find, getChar, live, moves, nulTerminate, rawAccess, setChar, toStream, and trim.
◆ RecordMemBlobSizeAtDestruct()
|
static |
Definition at line 27 of file Stats.cc.
References MemBlobSizeAtDestructRecorder.
Referenced by MemBlob::~MemBlob().
◆ RecordSBufSizeAtDestruct()
|
static |
Definition at line 20 of file Stats.cc.
References SBufSizeAtDestructRecorder.
Referenced by SBuf::~SBuf().
Member Data Documentation
◆ alloc
uint64_t SBufStats::alloc = 0 |
Definition at line 36 of file Stats.h.
Referenced by SBuf::SBuf(), dump(), and operator+=().
◆ allocCopy
uint64_t SBufStats::allocCopy = 0 |
Definition at line 37 of file Stats.h.
Referenced by SBuf::SBuf(), dump(), and operator+=().
◆ allocFromCString
uint64_t SBufStats::allocFromCString = 0 |
Definition at line 38 of file Stats.h.
Referenced by SBuf::SBuf(), dump(), and operator+=().
◆ append
uint64_t SBufStats::append = 0 |
Definition at line 41 of file Stats.h.
Referenced by dump(), SBuf::lowAppend(), operator+=(), and SBuf::vappendf().
◆ assignFast
uint64_t SBufStats::assignFast = 0 |
Definition at line 39 of file Stats.h.
Referenced by SBuf::assign(), dump(), and operator+=().
◆ caseChange
uint64_t SBufStats::caseChange = 0 |
Definition at line 54 of file Stats.h.
Referenced by dump(), operator+=(), SBuf::toLower(), and SBuf::toUpper().
◆ chop
uint64_t SBufStats::chop = 0 |
Definition at line 51 of file Stats.h.
Referenced by SBuf::chop(), dump(), and operator+=().
◆ clear
uint64_t SBufStats::clear = 0 |
Definition at line 40 of file Stats.h.
Referenced by SBuf::clear(), dump(), and operator+=().
◆ compareFast
uint64_t SBufStats::compareFast = 0 |
Definition at line 47 of file Stats.h.
Referenced by SBuf::compare(), dump(), operator+=(), SBuf::operator==(), and SBuf::startsWith().
◆ compareSlow
uint64_t SBufStats::compareSlow = 0 |
Definition at line 46 of file Stats.h.
Referenced by SBuf::compare(), dump(), operator+=(), and SBuf::operator==().
◆ copyOut
uint64_t SBufStats::copyOut = 0 |
Definition at line 48 of file Stats.h.
Referenced by SBuf::copy(), dump(), and operator+=().
◆ cowAllocCopy
uint64_t SBufStats::cowAllocCopy = 0 |
Definition at line 58 of file Stats.h.
Referenced by dump(), operator+=(), and SBuf::reAlloc().
◆ cowAvoided
uint64_t SBufStats::cowAvoided = 0 |
Definition at line 55 of file Stats.h.
Referenced by SBuf::cow(), dump(), and operator+=().
◆ cowJustAlloc
uint64_t SBufStats::cowJustAlloc = 0 |
Definition at line 57 of file Stats.h.
Referenced by dump(), operator+=(), and SBuf::reAlloc().
◆ cowShift
uint64_t SBufStats::cowShift = 0 |
Definition at line 56 of file Stats.h.
Referenced by SBuf::cow(), dump(), and operator+=().
◆ find
uint64_t SBufStats::find = 0 |
Definition at line 53 of file Stats.h.
Referenced by dump(), SBuf::find(), SBuf::findFirstNotOf(), SBuf::findFirstOf(), SBuf::findLastNotOf(), SBuf::findLastOf(), operator+=(), and SBuf::rfind().
◆ getChar
uint64_t SBufStats::getChar = 0 |
Definition at line 45 of file Stats.h.
Referenced by dump(), operator+=(), and SBuf::operator[]().
◆ live
uint64_t SBufStats::live = 0 |
Definition at line 59 of file Stats.h.
Referenced by SBuf::SBuf(), SBuf::~SBuf(), dump(), and operator+=().
◆ MemBlobSizeAtDestructRecorder
|
static |
Definition at line 66 of file Stats.h.
Referenced by RecordMemBlobSizeAtDestruct(), and SBufStatsAction::RegisterWithCacheManager().
◆ moves
uint64_t SBufStats::moves = 0 |
Definition at line 42 of file Stats.h.
Referenced by SBuf::SBuf(), dump(), operator+=(), and SBuf::operator=().
◆ nulTerminate
uint64_t SBufStats::nulTerminate = 0 |
Definition at line 50 of file Stats.h.
Referenced by SBuf::c_str(), dump(), and operator+=().
◆ rawAccess
uint64_t SBufStats::rawAccess = 0 |
Definition at line 49 of file Stats.h.
Referenced by SBuf::c_str(), dump(), operator+=(), SBuf::rawContent(), and SBuf::rawSpace().
◆ SBufSizeAtDestructRecorder
|
static |
Definition at line 64 of file Stats.h.
Referenced by RecordSBufSizeAtDestruct(), and SBufStatsAction::RegisterWithCacheManager().
◆ setChar
uint64_t SBufStats::setChar = 0 |
Definition at line 44 of file Stats.h.
Referenced by SBuf::c_str(), dump(), operator+=(), and SBuf::setAt().
◆ toStream
uint64_t SBufStats::toStream = 0 |
Definition at line 43 of file Stats.h.
Referenced by dump(), operator+=(), and SBuf::print().
◆ trim
uint64_t SBufStats::trim = 0 |
Definition at line 52 of file Stats.h.
Referenced by dump(), operator+=(), and SBuf::trim().
The documentation for this class was generated from the following files:
- src/sbuf/Stats.h
- src/sbuf/Stats.cc
- src/tests/stub_SBuf.cc