#include <splay.h>
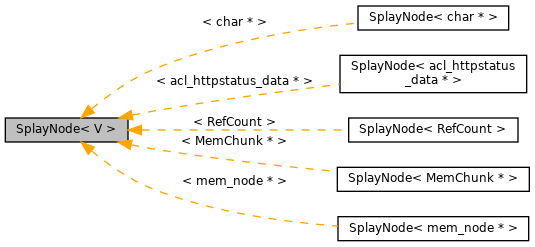
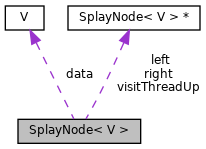
Public Types | |
typedef V | Value |
typedef int | SPLAYCMP(Value const &a, Value const &b) |
Public Member Functions | |
SplayNode (Value const &) | |
SplayNode< V > const * | start () const |
SplayNode< V > const * | finish () const |
SplayNode< V > * | remove (const Value data, SPLAYCMP *compare) |
SplayNode< V > * | insert (Value data, SPLAYCMP *compare) |
template<class FindValue > | |
SplayNode< V > * | splay (const FindValue &data, int(*compare)(FindValue const &a, Value const &b)) const |
Public Attributes | |
Value | data |
SplayNode< V > * | left |
SplayNode< V > * | right |
SplayNode< V > * | visitThreadUp |
Detailed Description
Member Typedef Documentation
◆ SPLAYCMP
◆ Value
Constructor & Destructor Documentation
◆ SplayNode()
SplayNode< V >::SplayNode | ( | Value const & | someData | ) |
Member Function Documentation
◆ finish()
◆ insert()
Definition at line 145 of file splay.h.
References SplayNode< V >::left, SplayNode< V >::right, and splayLastResult.
◆ remove()
Definition at line 120 of file splay.h.
References SplayNode< V >::left, SplayNode< V >::right, and splayLastResult.
◆ splay()
SplayNode< V > * SplayNode< V >::splay | ( | const FindValue & | data, |
int(*)(FindValue const &a, Value const &b) | compare | ||
) | const |
look in the splay for data for where compare(data,candidate) == true. return NULL if not found, a pointer to the sought data if found.
Definition at line 171 of file splay.h.
References SplayNode< V >::data, SplayNode< V >::left, SplayNode< V >::right, and splayLastResult.
◆ start()
Member Data Documentation
◆ data
Definition at line 25 of file splay.h.
Referenced by mem_hdr::endOffset(), mem_hdr::freeDataUpto(), mem_hdr::lowestOffset(), mem_hdr::nodeToRecieve(), SplayNode< V >::splay(), and testSplayOfNodes().
◆ left
Definition at line 26 of file splay.h.
Referenced by SplayConstIterator< V >::addLeftPath(), SplayNode< V >::insert(), SplayNode< V >::remove(), and SplayNode< V >::splay().
◆ right
Definition at line 27 of file splay.h.
Referenced by SplayConstIterator< V >::advance(), SplayNode< V >::insert(), SplayNode< V >::remove(), and SplayNode< V >::splay().
◆ visitThreadUp
The documentation for this class was generated from the following file:
- include/splay.h