#include <Controlled.h>
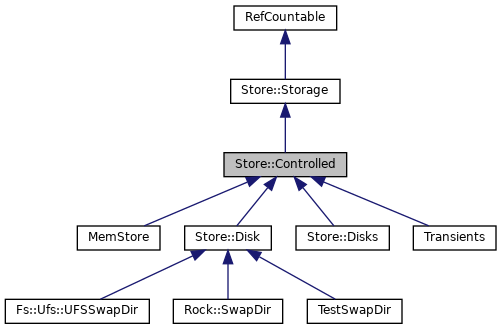
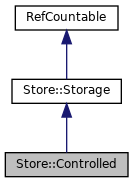
Public Member Functions | |
virtual StoreEntry * | get (const cache_key *)=0 |
virtual void | reference (StoreEntry &e)=0 |
somebody needs this entry (many cache replacement policies need to know) More... | |
virtual bool | dereference (StoreEntry &e)=0 |
virtual void | updateHeaders (StoreEntry *) |
make stored metadata and HTTP headers the same as in the given entry More... | |
virtual bool | anchorToCache (StoreEntry &) |
virtual bool | updateAnchored (StoreEntry &) |
virtual void | create ()=0 |
create system resources needed for this store to operate in the future More... | |
virtual void | init ()=0 |
virtual uint64_t | maxSize () const =0 |
virtual uint64_t | minSize () const =0 |
the minimum size the store will shrink to via normal housekeeping More... | |
virtual uint64_t | currentSize () const =0 |
current size More... | |
virtual uint64_t | currentCount () const =0 |
the total number of objects stored right now More... | |
virtual int64_t | maxObjectSize () const =0 |
the maximum size of a storable object; -1 if unlimited More... | |
virtual void | getStats (StoreInfoStats &stats) const =0 |
collect statistics More... | |
virtual void | stat (StoreEntry &e) const =0 |
virtual void | evictCached (StoreEntry &e)=0 |
virtual void | evictIfFound (const cache_key *)=0 |
virtual int | callback () |
called once every main loop iteration; TODO: Move to UFS code. More... | |
virtual void | maintain ()=0 |
perform regular periodic maintenance; TODO: move to UFSSwapDir::Maintain More... | |
virtual void | sync () |
prepare for shutdown More... | |
Detailed Description
Storage controlled by a Controller. This API is shared among Disks, Disk, Memory caches and Transients.
Definition at line 18 of file Controlled.h.
Member Function Documentation
◆ anchorToCache()
|
inlinevirtual |
tie StoreEntry to this storage if this storage has a matching entry
- Return values
-
true if this storage has a matching entry
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 39 of file Controlled.h.
Referenced by Store::Disks::anchorToCache().
◆ callback()
|
inlinevirtualinherited |
Reimplemented in Fs::Ufs::UFSSwapDir, Store::Controller, Store::Disks, and StoreControllerStub.
◆ create()
|
pure virtualinherited |
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, MemStore, Store::Controller, Store::Disk, Store::Disks, and Transients.
◆ currentCount()
|
pure virtualinherited |
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, MemStore, Store::Controller, Store::Disks, StoreControllerStub, TestSwapDir, and Transients.
◆ currentSize()
|
pure virtualinherited |
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, MemStore, Store::Controller, Store::Disks, StoreControllerStub, TestSwapDir, and Transients.
◆ dereference()
|
pure virtual |
somebody no longer needs this entry (usually after calling reference()) return false iff the idle entry should be destroyed
Implemented in Fs::Ufs::UFSSwapDir, Rock::SwapDir, MemStore, Store::Disk, Store::Disks, and Transients.
◆ evictCached()
|
pure virtualinherited |
Prevent new get() calls from returning the matching entry. If the matching entry is unused, it may be removed from the store now. The store entry is matched using either e
attachment info or e.key
.
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, MemStore, Store::Controller, Store::Disks, TestSwapDir, and Transients.
Referenced by Store::Disks::evictCached().
◆ evictIfFound()
|
pure virtualinherited |
An evictCached() equivalent for callers that did not get() a StoreEntry. Callers with StoreEntry objects must use evictCached() instead.
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, MemStore, Store::Controller, Store::Disks, TestSwapDir, and Transients.
◆ get()
|
pure virtual |
- Returns
- a possibly unlocked/unregistered stored entry with key (or nil) The returned entry might not match the caller's Store ID or method. The caller must abandon()/release() the entry or register it with Root(). This method must not trigger slow I/O operations (e.g., disk swap in).
Implemented in MemStore, Store::Disk, Store::Disks, Transients, and Rock::SwapDir.
◆ getStats()
|
pure virtualinherited |
Implemented in StoreControllerStub, MemStore, Store::Controller, Store::Disk, Store::Disks, and Transients.
◆ init()
|
pure virtualinherited |
Start preparing the store for use. To check readiness, callers should use readable() and writable() methods.
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, MemStore, Store::Controller, Store::Disks, StoreControllerStub, TestSwapDir, and Transients.
◆ maintain()
|
pure virtualinherited |
Implemented in Rock::SwapDir, Fs::Ufs::UFSSwapDir, MemStore, Store::Controller, Store::Disk, Store::Disks, StoreControllerStub, and Transients.
◆ maxObjectSize()
|
pure virtualinherited |
Implemented in MemStore, Store::Controller, Store::Disk, Store::Disks, StoreControllerStub, and Transients.
◆ maxSize()
|
pure virtualinherited |
The maximum size the store will support in normal use. Inaccuracy is permitted, but may throw estimates for memory etc out of whack.
Implemented in MemStore, Store::Controller, Store::Disk, Store::Disks, StoreControllerStub, TestSwapDir, and Transients.
◆ minSize()
|
pure virtualinherited |
Implemented in MemStore, Store::Controller, Store::Disk, Store::Disks, StoreControllerStub, and Transients.
◆ reference()
|
pure virtual |
Implemented in Fs::Ufs::UFSSwapDir, Store::Disks, Rock::SwapDir, MemStore, Store::Disk, and Transients.
◆ stat()
|
pure virtualinherited |
Output stats to the provided store entry. TODO: make these calls asynchronous
Implemented in Store::Controller, Store::Disk, Store::Disks, StoreControllerStub, TestSwapDir, MemStore, and Transients.
◆ sync()
|
inlinevirtualinherited |
Reimplemented in Fs::Ufs::UFSSwapDir, Store::Controller, and Store::Disks.
◆ updateAnchored()
|
inlinevirtual |
Update a local Transients entry with fresh info from this cache (if any). Return true iff the cache supports Transients entries and the given local Transients entry is now in sync with this storage.
Reimplemented in Rock::SwapDir, MemStore, and Store::Disks.
Definition at line 44 of file Controlled.h.
Referenced by Store::Disks::updateAnchored().
◆ updateHeaders()
|
inlinevirtual |
Reimplemented in Store::Disks, Rock::SwapDir, and MemStore.
Definition at line 35 of file Controlled.h.
Referenced by Store::Disks::updateHeaders().
The documentation for this class was generated from the following file:
- src/store/Controlled.h