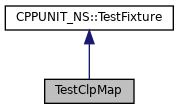
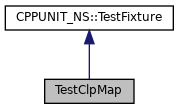
Protected Types | |
using | Map = ClpMap< std::string, int > |
Protected Member Functions | |
void | testMemoryCounter () |
void | testConstructor () |
void | testEntryCounter () |
void | testPutGetDelete () |
void | testMisses () |
void | testMemoryLimit () |
void | testTtlExpiration () |
void | testReplaceEntryWithShorterTtl () |
void | testZeroTtl () |
void | testNegativeTtl () |
void | testPurgeIsLru () |
void | testClassicLoopTraversal () |
void | testRangeLoopTraversal () |
void | addSequenceOfEntriesToMap (Map &, size_t count, Map::mapped_type startWith, Map::Ttl) |
void | fillMapWithEntries (Map &) |
add (more than) enough entries to make the map full More... | |
void | addOneEntry (Map &, Map::mapped_type) |
void | addOneEntry (Map &, Map::mapped_type, Map::Ttl) |
Detailed Description
Definition at line 17 of file testClpMap.cc.
Member Typedef Documentation
◆ Map
|
protected |
Definition at line 36 of file testClpMap.cc.
Member Function Documentation
◆ addOneEntry() [1/2]
|
protected |
generate and add an entry with a given value (and a matching key) to the map using map-default TTL
Definition at line 87 of file testClpMap.cc.
References ClpMap< Key, Value, MemoryUsedBy >::add(), and ClpMap< Key, Value, MemoryUsedBy >::get().
Referenced by testNegativeTtl(), testPurgeIsLru(), testReplaceEntryWithShorterTtl(), testTtlExpiration(), and testZeroTtl().
◆ addOneEntry() [2/2]
|
protected |
generate and add an entry with a given value, a matching key, and a given TTL to the map
Definition at line 96 of file testClpMap.cc.
References ClpMap< Key, Value, MemoryUsedBy >::add(), and ClpMap< Key, Value, MemoryUsedBy >::get().
◆ addSequenceOfEntriesToMap()
|
protected |
Generate and insert the given number of entries into the given map. Each entry is guaranteed to be inserted, but that insertion may purge other entries, including entries previously added during the same method call.
Definition at line 74 of file testClpMap.cc.
References ClpMap< Key, Value, MemoryUsedBy >::add().
Referenced by fillMapWithEntries(), testClassicLoopTraversal(), testEntryCounter(), testPutGetDelete(), and testRangeLoopTraversal().
◆ CPPUNIT_TEST() [1/13]
|
private |
◆ CPPUNIT_TEST() [2/13]
|
private |
◆ CPPUNIT_TEST() [3/13]
|
private |
◆ CPPUNIT_TEST() [4/13]
|
private |
◆ CPPUNIT_TEST() [5/13]
|
private |
◆ CPPUNIT_TEST() [6/13]
|
private |
◆ CPPUNIT_TEST() [7/13]
|
private |
◆ CPPUNIT_TEST() [8/13]
|
private |
◆ CPPUNIT_TEST() [9/13]
|
private |
◆ CPPUNIT_TEST() [10/13]
|
private |
◆ CPPUNIT_TEST() [11/13]
|
private |
◆ CPPUNIT_TEST() [12/13]
|
private |
◆ CPPUNIT_TEST() [13/13]
|
private |
◆ CPPUNIT_TEST_SUITE()
|
private |
◆ CPPUNIT_TEST_SUITE_END()
|
private |
◆ fillMapWithEntries()
|
protected |
Definition at line 81 of file testClpMap.cc.
References addSequenceOfEntriesToMap(), and ClpMap< Key, Value, MemoryUsedBy >::memLimit().
Referenced by testMemoryLimit(), testMisses(), and testPurgeIsLru().
◆ testClassicLoopTraversal()
|
protected |
Definition at line 352 of file testClpMap.cc.
References addSequenceOfEntriesToMap(), ClpMap< Key, Value, MemoryUsedBy >::cbegin(), and ClpMap< Key, Value, MemoryUsedBy >::cend().
◆ testConstructor()
|
protected |
Definition at line 161 of file testClpMap.cc.
References ClpMap< Key, Value, MemoryUsedBy >::entries(), ClpMap< Key, Value, MemoryUsedBy >::freeMem(), ClpMap< Key, Value, MemoryUsedBy >::memLimit(), and ClpMap< Key, Value, MemoryUsedBy >::memoryUsed().
◆ testEntryCounter()
|
protected |
Definition at line 132 of file testClpMap.cc.
References ClpMap< Key, Value, MemoryUsedBy >::add(), addSequenceOfEntriesToMap(), and ClpMap< Key, Value, MemoryUsedBy >::entries().
◆ testMemoryCounter()
|
protected |
Definition at line 150 of file testClpMap.cc.
References DefaultMemoryUsage().
◆ testMemoryLimit()
|
protected |
Definition at line 189 of file testClpMap.cc.
References ClpMap< Key, Value, MemoryUsedBy >::entries(), fillMapWithEntries(), ClpMap< Key, Value, MemoryUsedBy >::memoryUsed(), and ClpMap< Key, Value, MemoryUsedBy >::setMemLimit().
◆ testMisses()
|
protected |
Definition at line 121 of file testClpMap.cc.
References ClpMap< Key, Value, MemoryUsedBy >::del(), ClpMap< Key, Value, MemoryUsedBy >::entries(), fillMapWithEntries(), and ClpMap< Key, Value, MemoryUsedBy >::get().
◆ testNegativeTtl()
|
protected |
Definition at line 312 of file testClpMap.cc.
References ClpMap< Key, Value, MemoryUsedBy >::add(), addOneEntry(), and ClpMap< Key, Value, MemoryUsedBy >::get().
◆ testPurgeIsLru()
|
protected |
Definition at line 331 of file testClpMap.cc.
References addOneEntry(), fillMapWithEntries(), and ClpMap< Key, Value, MemoryUsedBy >::get().
◆ testPutGetDelete()
|
protected |
Definition at line 105 of file testClpMap.cc.
References ClpMap< Key, Value, MemoryUsedBy >::add(), addSequenceOfEntriesToMap(), ClpMap< Key, Value, MemoryUsedBy >::del(), and ClpMap< Key, Value, MemoryUsedBy >::get().
◆ testRangeLoopTraversal()
|
protected |
Definition at line 367 of file testClpMap.cc.
References addSequenceOfEntriesToMap().
◆ testReplaceEntryWithShorterTtl()
|
protected |
Definition at line 267 of file testClpMap.cc.
References addOneEntry(), ClpMap< Key, Value, MemoryUsedBy >::get(), and squid_curtime.
◆ testTtlExpiration()
|
protected |
Definition at line 234 of file testClpMap.cc.
References addOneEntry(), ClpMap< Key, Value, MemoryUsedBy >::get(), and squid_curtime.
◆ testZeroTtl()
|
protected |
Definition at line 285 of file testClpMap.cc.
References addOneEntry(), ClpMap< Key, Value, MemoryUsedBy >::get(), and squid_curtime.
The documentation for this class was generated from the following file:
- src/tests/testClpMap.cc