#include <Sequence.h>
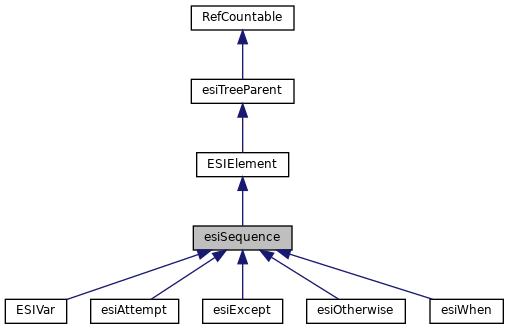
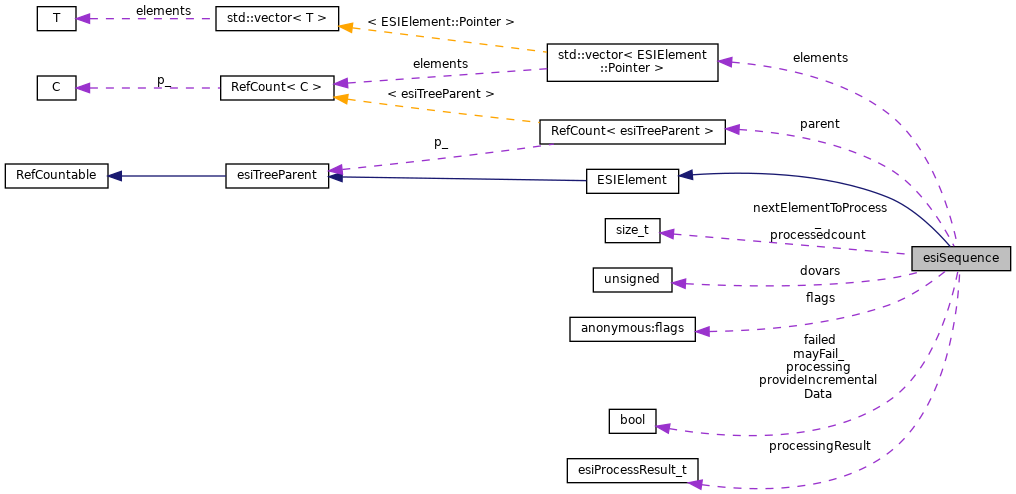
Public Types | |
enum | ESIElementType_t { ESI_ELEMENT_NONE , ESI_ELEMENT_INCLUDE , ESI_ELEMENT_COMMENT , ESI_ELEMENT_REMOVE , ESI_ELEMENT_TRY , ESI_ELEMENT_ATTEMPT , ESI_ELEMENT_EXCEPT , ESI_ELEMENT_VARS , ESI_ELEMENT_CHOOSE , ESI_ELEMENT_WHEN , ESI_ELEMENT_OTHERWISE , ESI_ELEMENT_ASSIGN } |
typedef RefCount< ESIElement > | Pointer |
Public Member Functions | |
esiSequence (esiTreeParentPtr, bool=false) | |
~esiSequence () override | |
void | render (ESISegment::Pointer) override |
bool | addElement (ESIElement::Pointer) override |
esiProcessResult_t | process (int dovars) override |
void | provideData (ESISegment::Pointer, ESIElement *) override |
bool | mayFail () const override |
void | wontFail () |
void | fail (ESIElement *, char const *anError=nullptr) override |
void | makeCachableElements (esiSequence const &old) |
Pointer | makeCacheable () const override |
void | makeUsableElements (esiSequence const &old, ESIVarState &) |
Pointer | makeUsable (esiTreeParentPtr, ESIVarState &) const override |
void | finish () override |
Static Public Member Functions | |
static ESIElementType_t | IdentifyElement (const char *) |
Public Attributes | |
Esi::Elements | elements |
size_t | processedcount |
struct { | |
unsigned int dovars:1 | |
} | flags |
Protected Member Functions | |
esiSequence (esiSequence const &) | |
Protected Attributes | |
esiTreeParentPtr | parent |
Private Member Functions | |
MEMPROXY_CLASS (esiSequence) | |
int | elementIndex (ESIElement::Pointer anElement) const |
esiProcessResult_t | processOne (int, size_t) |
size_t | nextElementToProcess () const |
void | nextElementToProcess (size_t const &) |
bool | finishedProcessing () const |
void | processStep (int dovars) |
Private Attributes | |
bool | mayFail_ |
bool | failed |
bool const | provideIncrementalData |
bool | processing |
esiProcessResult_t | processingResult |
size_t | nextElementToProcess_ |
Detailed Description
Definition at line 19 of file Sequence.h.
Member Typedef Documentation
◆ Pointer
|
inherited |
Member Enumeration Documentation
◆ ESIElementType_t
|
inherited |
Constructor & Destructor Documentation
◆ esiSequence() [1/2]
esiSequence::esiSequence | ( | esiTreeParentPtr | aParent, |
bool | incrementalFlag = false |
||
) |
Definition at line 33 of file Sequence.cc.
References flags.
Referenced by makeCacheable(), and makeUsable().
◆ ~esiSequence()
|
override |
Definition at line 27 of file Sequence.cc.
References debugs, elements, and FinishAllElements().
◆ esiSequence() [2/2]
|
protected |
Definition at line 322 of file Sequence.cc.
Member Function Documentation
◆ addElement()
|
overridevirtual |
Reimplemented from ESIElement.
Definition at line 148 of file Sequence.cc.
References DBG_CRITICAL, debugs, elements, RefCount< C >::getRaw(), and ESISegment::ListTransfer().
Referenced by makeCachableElements(), and makeUsableElements().
◆ elementIndex()
|
private |
◆ fail()
|
overridevirtual |
Reimplemented from esiTreeParent.
Definition at line 307 of file Sequence.cc.
References debugs, elements, esiTreeParent::fail(), failed, FinishAllElements(), parent, and processing.
◆ finish()
|
overridevirtual |
Implements ESIElement.
Definition at line 104 of file Sequence.cc.
References debugs, elements, FinishAllElements(), and parent.
◆ finishedProcessing()
|
private |
Definition at line 60 of file Sequence.cc.
References elements, and nextElementToProcess().
Referenced by process().
◆ IdentifyElement()
|
staticinherited |
Definition at line 852 of file Esi.cc.
References assert, ESIElement::ESI_ELEMENT_ASSIGN, ESIElement::ESI_ELEMENT_ATTEMPT, ESIElement::ESI_ELEMENT_CHOOSE, ESIElement::ESI_ELEMENT_COMMENT, ESIElement::ESI_ELEMENT_EXCEPT, ESIElement::ESI_ELEMENT_INCLUDE, ESIElement::ESI_ELEMENT_NONE, ESIElement::ESI_ELEMENT_OTHERWISE, ESIElement::ESI_ELEMENT_REMOVE, ESIElement::ESI_ELEMENT_TRY, ESIElement::ESI_ELEMENT_VARS, and ESIElement::ESI_ELEMENT_WHEN.
Referenced by ESIContext::end(), and ESIContext::start().
◆ makeCachableElements()
void esiSequence::makeCachableElements | ( | esiSequence const & | old | ) |
Definition at line 336 of file Sequence.cc.
References addElement(), assert, elements, and RefCount< C >::getRaw().
Referenced by makeCacheable().
◆ makeCacheable()
|
overridevirtual |
Implements ESIElement.
Definition at line 358 of file Sequence.cc.
References esiSequence(), assert, debugs, elements, failed, RefCount< C >::getRaw(), makeCachableElements(), and processedcount.
◆ makeUsable()
|
overridevirtual |
Implements ESIElement.
Definition at line 377 of file Sequence.cc.
References esiSequence(), assert, debugs, elements, failed, makeUsableElements(), parent, and processedcount.
◆ makeUsableElements()
void esiSequence::makeUsableElements | ( | esiSequence const & | old, |
ESIVarState & | newVarState | ||
) |
Definition at line 347 of file Sequence.cc.
References addElement(), assert, elements, and RefCount< C >::getRaw().
Referenced by esiWhen::makeUsable(), and makeUsable().
◆ mayFail()
|
overridevirtual |
Reimplemented from ESIElement.
Definition at line 66 of file Sequence.cc.
◆ MEMPROXY_CLASS()
|
private |
◆ nextElementToProcess() [1/2]
|
private |
Definition at line 48 of file Sequence.cc.
References nextElementToProcess_.
Referenced by finishedProcessing(), process(), and processStep().
◆ nextElementToProcess() [2/2]
|
private |
Definition at line 54 of file Sequence.cc.
References nextElementToProcess_.
◆ process()
|
overridevirtual |
Reimplemented from ESIElement.
Definition at line 238 of file Sequence.cc.
References assert, debugs, dovars, elements, ESI_PROCESS_COMPLETE, ESI_PROCESS_FAILED, ESI_PROCESS_PENDING_WONTFAIL, ESISegmentFreeList(), failed, FinishAllElements(), finishedProcessing(), flags, RefCount< C >::getRaw(), ESISegment::len, ESISegment::next, nextElementToProcess(), parent, processedcount, processing, processingResult, processStep(), esiTreeParent::provideData(), provideIncrementalData, render(), and wontFail().
Referenced by processOne(), and provideData().
◆ processOne()
|
private |
Definition at line 200 of file Sequence.cc.
References debugs, dovars, elements, ESI_PROCESS_COMPLETE, ESI_PROCESS_FAILED, ESI_PROCESS_PENDING_MAYFAIL, ESI_PROCESS_PENDING_WONTFAIL, fatal(), process(), and processedcount.
Referenced by processStep().
◆ processStep()
|
private |
Definition at line 187 of file Sequence.cc.
References debugs, dovars, nextElementToProcess(), processingResult, and processOne().
Referenced by process().
◆ provideData()
|
overridevirtual |
Reimplemented from esiTreeParent.
Definition at line 112 of file Sequence.cc.
References assert, debugs, elementIndex(), elements, ESI_PROCESS_FAILED, FinishAnElement(), flags, RefCount< C >::getRaw(), process(), and processing.
◆ render()
|
overridevirtual |
Implements ESIElement.
Definition at line 82 of file Sequence.cc.
References assert, debugs, elements, FinishAnElement(), ESISegment::next, processedcount, and ESISegment::tail().
Referenced by process().
◆ wontFail()
void esiSequence::wontFail | ( | ) |
Member Data Documentation
◆ dovars
unsigned int esiSequence::dovars |
Definition at line 43 of file Sequence.h.
Referenced by esiSequence(), process(), processOne(), and processStep().
◆ elements
Esi::Elements esiSequence::elements |
Definition at line 39 of file Sequence.h.
Referenced by ~esiSequence(), addElement(), elementIndex(), fail(), finish(), finishedProcessing(), makeCachableElements(), makeCacheable(), makeUsable(), makeUsableElements(), process(), processOne(), provideData(), and render().
◆ failed
|
private |
Definition at line 54 of file Sequence.h.
Referenced by fail(), makeCacheable(), makeUsable(), mayFail(), process(), and wontFail().
◆
struct { ... } esiSequence::flags |
Referenced by esiSequence(), ESIVar::ESIVar(), process(), and provideData().
◆ mayFail_
|
private |
Definition at line 53 of file Sequence.h.
Referenced by mayFail(), and wontFail().
◆ nextElementToProcess_
|
private |
Definition at line 59 of file Sequence.h.
Referenced by nextElementToProcess().
◆ parent
|
protected |
Definition at line 49 of file Sequence.h.
Referenced by fail(), finish(), esiWhen::makeUsable(), makeUsable(), and process().
◆ processedcount
size_t esiSequence::processedcount |
Definition at line 40 of file Sequence.h.
Referenced by makeCacheable(), makeUsable(), process(), processOne(), and render().
◆ processing
|
private |
Definition at line 57 of file Sequence.h.
Referenced by fail(), process(), and provideData().
◆ processingResult
|
private |
Definition at line 58 of file Sequence.h.
Referenced by process(), and processStep().
◆ provideIncrementalData
|
private |
Definition at line 56 of file Sequence.h.
Referenced by process().
The documentation for this class was generated from the following files:
- src/esi/Sequence.h
- src/esi/Sequence.cc