#include <Queue.h>
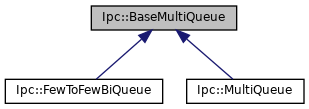
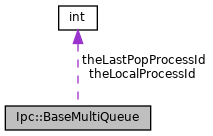
Public Member Functions | |
BaseMultiQueue (const int aLocalProcessId) | |
virtual | ~BaseMultiQueue () |
void | clearReaderSignal (const int remoteProcessId) |
clears the reader notification received by the local process from the remote process More... | |
void | clearAllReaderSignals () |
clears all reader notifications received by the local process More... | |
template<class Value > | |
bool | pop (int &remoteProcessId, Value &value) |
picks a process and calls OneToOneUniQueue::pop() using its queue More... | |
template<class Value > | |
bool | push (const int remoteProcessId, const Value &value) |
calls OneToOneUniQueue::push() using the given process queue More... | |
template<class Value > | |
bool | peek (int &remoteProcessId, Value &value) const |
peeks at the item likely to be pop()ed next More... | |
template<class Value > | |
void | stat (std::ostream &) const |
prints current state; suitable for cache manager reports More... | |
QueueReader::Balance & | localBalance () |
returns local reader's balance More... | |
const QueueReader::Balance & | balance (const int remoteProcessId) const |
returns reader's balance for a given remote process More... | |
QueueReader::Rate & | localRateLimit () |
returns local reader's rate limit More... | |
const QueueReader::Rate & | rateLimit (const int remoteProcessId) const |
returns reader's rate limit for a given remote process More... | |
int | inSize (const int remoteProcessId) const |
number of items in incoming queue from a given remote process More... | |
int | outSize (const int remoteProcessId) const |
number of items in outgoing queue to a given remote process More... | |
Protected Member Functions | |
virtual const OneToOneUniQueue & | inQueue (const int remoteProcessId) const =0 |
incoming queue from a given remote process More... | |
OneToOneUniQueue & | inQueue (const int remoteProcessId) |
virtual const OneToOneUniQueue & | outQueue (const int remoteProcessId) const =0 |
outgoing queue to a given remote process More... | |
OneToOneUniQueue & | outQueue (const int remoteProcessId) |
virtual const QueueReader & | localReader () const =0 |
QueueReader & | localReader () |
virtual const QueueReader & | remoteReader (const int remoteProcessId) const =0 |
QueueReader & | remoteReader (const int remoteProcessId) |
virtual int | remotesCount () const =0 |
virtual int | remotesIdOffset () const =0 |
Protected Attributes | |
const int | theLocalProcessId |
process ID of this queue More... | |
Private Attributes | |
int | theLastPopProcessId |
the ID of the last process we tried to pop() from More... | |
Detailed Description
Base class for lockless fixed-capacity bidirectional queues for a limited number processes.
Constructor & Destructor Documentation
◆ BaseMultiQueue()
Ipc::BaseMultiQueue::BaseMultiQueue | ( | const int | aLocalProcessId | ) |
◆ ~BaseMultiQueue()
|
inlinevirtual |
Member Function Documentation
◆ balance()
const Ipc::QueueReader::Balance & Ipc::BaseMultiQueue::balance | ( | const int | remoteProcessId | ) | const |
Definition at line 180 of file Queue.cc.
References Ipc::QueueReader::balance.
◆ clearAllReaderSignals()
void Ipc::BaseMultiQueue::clearAllReaderSignals | ( | ) |
Definition at line 172 of file Queue.cc.
References Ipc::QueueReader::clearSignal(), debugs, and Ipc::QueueReader::id.
◆ clearReaderSignal()
void Ipc::BaseMultiQueue::clearReaderSignal | ( | const int | remoteProcessId | ) |
◆ inQueue() [1/2]
|
protected |
◆ inQueue() [2/2]
|
protectedpure virtual |
Implemented in Ipc::FewToFewBiQueue, and Ipc::MultiQueue.
◆ inSize()
Definition at line 204 of file Queue.h.
References inQueue(), and Ipc::OneToOneUniQueue::size().
◆ localBalance()
|
inline |
Definition at line 192 of file Queue.h.
References Ipc::QueueReader::balance, and localReader().
◆ localRateLimit()
|
inline |
Definition at line 198 of file Queue.h.
References localReader(), and Ipc::QueueReader::rateLimit.
◆ localReader() [1/2]
|
protected |
◆ localReader() [2/2]
|
protectedpure virtual |
Implemented in Ipc::FewToFewBiQueue, and Ipc::MultiQueue.
Referenced by localBalance(), localRateLimit(), pop(), and stat().
◆ outQueue() [1/2]
|
protected |
◆ outQueue() [2/2]
|
protectedpure virtual |
Implemented in Ipc::FewToFewBiQueue, and Ipc::MultiQueue.
◆ outSize()
Definition at line 207 of file Queue.h.
References outQueue(), and Ipc::OneToOneUniQueue::size().
◆ peek()
bool Ipc::BaseMultiQueue::peek | ( | int & | remoteProcessId, |
Value & | value | ||
) | const |
Definition at line 568 of file Queue.h.
References inQueue(), Ipc::OneToOneUniQueue::peek(), remotesCount(), remotesIdOffset(), and theLastPopProcessId.
◆ pop()
Definition at line 540 of file Queue.h.
References debugs, inQueue(), localReader(), Ipc::OneToOneUniQueue::pop(), remotesCount(), remotesIdOffset(), Ipc::OneToOneUniQueue::size(), theLastPopProcessId, and theLocalProcessId.
◆ push()
bool Ipc::BaseMultiQueue::push | ( | const int | remoteProcessId, |
const Value & | value | ||
) |
Definition at line 558 of file Queue.h.
References debugs, outQueue(), Ipc::OneToOneUniQueue::push(), remoteReader(), Ipc::OneToOneUniQueue::size(), and theLocalProcessId.
◆ rateLimit()
const Ipc::QueueReader::Rate & Ipc::BaseMultiQueue::rateLimit | ( | const int | remoteProcessId | ) | const |
Definition at line 187 of file Queue.cc.
References Ipc::QueueReader::rateLimit.
◆ remoteReader() [1/2]
|
protected |
◆ remoteReader() [2/2]
|
protectedpure virtual |
Implemented in Ipc::FewToFewBiQueue, and Ipc::MultiQueue.
Referenced by push().
◆ remotesCount()
|
protectedpure virtual |
Implemented in Ipc::FewToFewBiQueue, and Ipc::MultiQueue.
◆ remotesIdOffset()
|
protectedpure virtual |
Implemented in Ipc::FewToFewBiQueue, and Ipc::MultiQueue.
◆ stat()
void Ipc::BaseMultiQueue::stat | ( | std::ostream & | os | ) | const |
Definition at line 586 of file Queue.h.
References inQueue(), localReader(), outQueue(), remotesCount(), remotesIdOffset(), and theLocalProcessId.
Member Data Documentation
◆ theLastPopProcessId
|
private |
◆ theLocalProcessId
|
protected |
The documentation for this class was generated from the following files: