#include <Queue.h>
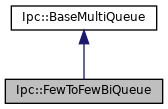
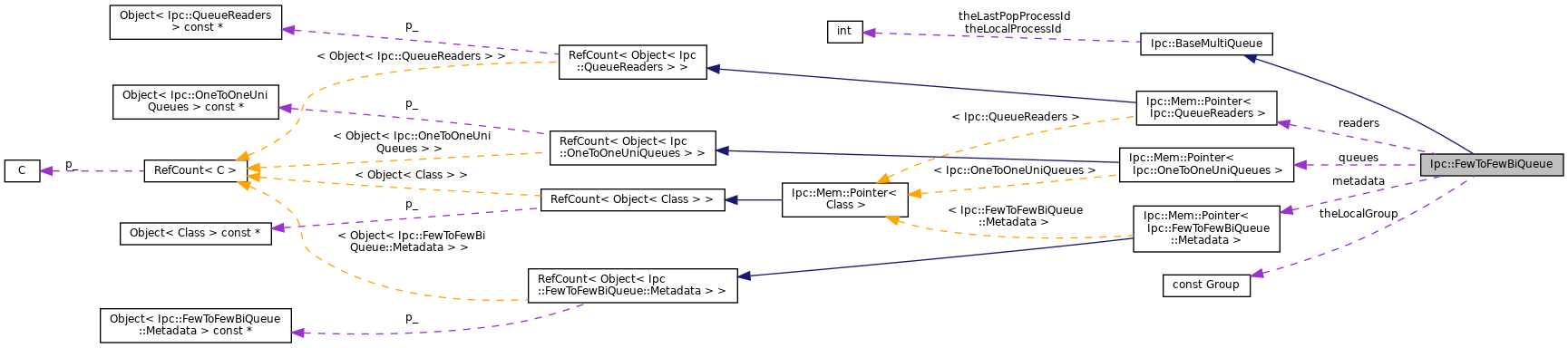
Classes | |
struct | Metadata |
Shared metadata for FewToFewBiQueue. More... | |
class | Owner |
Public Types | |
enum | Group { groupA = 0 , groupB = 1 } |
typedef OneToOneUniQueue::Full | Full |
typedef OneToOneUniQueue::ItemTooLarge | ItemTooLarge |
Public Member Functions | |
FewToFewBiQueue (const String &id, const Group aLocalGroup, const int aLocalProcessId) | |
template<class Value > | |
bool | findOldest (const int remoteProcessId, Value &value) const |
void | clearReaderSignal (const int remoteProcessId) |
clears the reader notification received by the local process from the remote process More... | |
void | clearAllReaderSignals () |
clears all reader notifications received by the local process More... | |
template<class Value > | |
bool | pop (int &remoteProcessId, Value &value) |
picks a process and calls OneToOneUniQueue::pop() using its queue More... | |
template<class Value > | |
bool | push (const int remoteProcessId, const Value &value) |
calls OneToOneUniQueue::push() using the given process queue More... | |
template<class Value > | |
bool | peek (int &remoteProcessId, Value &value) const |
peeks at the item likely to be pop()ed next More... | |
template<class Value > | |
void | stat (std::ostream &) const |
prints current state; suitable for cache manager reports More... | |
QueueReader::Balance & | localBalance () |
returns local reader's balance More... | |
const QueueReader::Balance & | balance (const int remoteProcessId) const |
returns reader's balance for a given remote process More... | |
QueueReader::Rate & | localRateLimit () |
returns local reader's rate limit More... | |
const QueueReader::Rate & | rateLimit (const int remoteProcessId) const |
returns reader's rate limit for a given remote process More... | |
int | inSize (const int remoteProcessId) const |
number of items in incoming queue from a given remote process More... | |
int | outSize (const int remoteProcessId) const |
number of items in outgoing queue to a given remote process More... | |
Static Public Member Functions | |
static Owner * | Init (const String &id, const int groupASize, const int groupAIdOffset, const int groupBSize, const int groupBIdOffset, const unsigned int maxItemSize, const int capacity) |
static int | MaxItemsCount (const int groupASize, const int groupBSize, const int capacity) |
maximum number of items in the queue More... | |
Protected Member Functions | |
const OneToOneUniQueue & | inQueue (const int remoteProcessId) const override |
incoming queue from a given remote process More... | |
const OneToOneUniQueue & | outQueue (const int remoteProcessId) const override |
outgoing queue to a given remote process More... | |
const QueueReader & | localReader () const override |
const QueueReader & | remoteReader (const int processId) const override |
int | remotesCount () const override |
int | remotesIdOffset () const override |
OneToOneUniQueue & | inQueue (const int remoteProcessId) |
OneToOneUniQueue & | outQueue (const int remoteProcessId) |
QueueReader & | localReader () |
QueueReader & | remoteReader (const int remoteProcessId) |
Protected Attributes | |
const int | theLocalProcessId |
process ID of this queue More... | |
Private Member Functions | |
bool | validProcessId (const Group group, const int processId) const |
int | oneToOneQueueIndex (const Group fromGroup, const int fromProcessId, const Group toGroup, const int toProcessId) const |
const OneToOneUniQueue & | oneToOneQueue (const Group fromGroup, const int fromProcessId, const Group toGroup, const int toProcessId) const |
int | readerIndex (const Group group, const int processId) const |
Group | localGroup () const |
Group | remoteGroup () const |
Private Attributes | |
const Mem::Pointer< Metadata > | metadata |
shared metadata More... | |
const Mem::Pointer< OneToOneUniQueues > | queues |
unidirection one-to-one queues More... | |
const Mem::Pointer< QueueReaders > | readers |
readers array More... | |
const Group | theLocalGroup |
group of this queue More... | |
int | theLastPopProcessId |
the ID of the last process we tried to pop() from More... | |
Detailed Description
Lockless fixed-capacity bidirectional queue for a limited number processes. Allows communication between two groups of processes: any process in one group may send data to and receive from any process in another group, but processes in the same group can not communicate. Process in each group has a unique integer ID in [groupIdOffset, groupIdOffset + groupSize) range.
Member Typedef Documentation
◆ Full
◆ ItemTooLarge
Member Enumeration Documentation
◆ Group
Constructor & Destructor Documentation
◆ FewToFewBiQueue()
Member Function Documentation
◆ balance()
|
inherited |
Definition at line 180 of file Queue.cc.
References Ipc::QueueReader::balance.
◆ clearAllReaderSignals()
|
inherited |
Definition at line 172 of file Queue.cc.
References Ipc::QueueReader::clearSignal(), debugs, and Ipc::QueueReader::id.
◆ clearReaderSignal()
|
inherited |
◆ findOldest()
bool Ipc::FewToFewBiQueue::findOldest | ( | const int | remoteProcessId, |
Value & | value | ||
) | const |
finds the oldest item in incoming and outgoing queues between us and the given remote process
Definition at line 611 of file Queue.h.
References debugs, inQueue(), outQueue(), Ipc::OneToOneUniQueue::peek(), remoteGroup(), Ipc::OneToOneUniQueue::size(), Ipc::BaseMultiQueue::theLocalProcessId, and validProcessId().
◆ Init()
|
static |
Definition at line 228 of file Queue.cc.
Referenced by IpcIoRr::create().
◆ inQueue() [1/2]
|
protectedinherited |
◆ inQueue() [2/2]
|
overrideprotectedvirtual |
Implements Ipc::BaseMultiQueue.
Definition at line 295 of file Queue.cc.
Referenced by findOldest().
◆ inSize()
Definition at line 204 of file Queue.h.
References Ipc::BaseMultiQueue::inQueue(), and Ipc::OneToOneUniQueue::size().
◆ localBalance()
|
inlineinherited |
Definition at line 192 of file Queue.h.
References Ipc::QueueReader::balance, and Ipc::BaseMultiQueue::localReader().
◆ localGroup()
|
inlineprivate |
Definition at line 299 of file Queue.h.
References theLocalGroup.
◆ localRateLimit()
|
inlineinherited |
Definition at line 198 of file Queue.h.
References Ipc::BaseMultiQueue::localReader(), and Ipc::QueueReader::rateLimit.
◆ localReader() [1/2]
|
protectedinherited |
◆ localReader() [2/2]
|
overrideprotectedvirtual |
Implements Ipc::BaseMultiQueue.
Definition at line 318 of file Queue.cc.
Referenced by FewToFewBiQueue().
◆ MaxItemsCount()
|
static |
Definition at line 247 of file Queue.cc.
Referenced by IpcIoRr::claimMemoryNeeds().
◆ oneToOneQueue()
|
private |
◆ oneToOneQueueIndex()
◆ outQueue() [1/2]
|
protectedinherited |
◆ outQueue() [2/2]
|
overrideprotectedvirtual |
Implements Ipc::BaseMultiQueue.
Definition at line 302 of file Queue.cc.
Referenced by findOldest().
◆ outSize()
Definition at line 207 of file Queue.h.
References Ipc::BaseMultiQueue::outQueue(), and Ipc::OneToOneUniQueue::size().
◆ peek()
|
inherited |
Definition at line 568 of file Queue.h.
References Ipc::BaseMultiQueue::inQueue(), Ipc::OneToOneUniQueue::peek(), Ipc::BaseMultiQueue::remotesCount(), Ipc::BaseMultiQueue::remotesIdOffset(), and Ipc::BaseMultiQueue::theLastPopProcessId.
◆ pop()
|
inherited |
Definition at line 540 of file Queue.h.
References debugs, Ipc::BaseMultiQueue::inQueue(), Ipc::BaseMultiQueue::localReader(), Ipc::OneToOneUniQueue::pop(), Ipc::BaseMultiQueue::remotesCount(), Ipc::BaseMultiQueue::remotesIdOffset(), Ipc::OneToOneUniQueue::size(), Ipc::BaseMultiQueue::theLastPopProcessId, and Ipc::BaseMultiQueue::theLocalProcessId.
◆ push()
|
inherited |
Definition at line 558 of file Queue.h.
References debugs, Ipc::BaseMultiQueue::outQueue(), Ipc::OneToOneUniQueue::push(), Ipc::BaseMultiQueue::remoteReader(), Ipc::OneToOneUniQueue::size(), and Ipc::BaseMultiQueue::theLocalProcessId.
◆ rateLimit()
|
inherited |
Definition at line 187 of file Queue.cc.
References Ipc::QueueReader::rateLimit.
◆ readerIndex()
◆ remoteGroup()
|
inlineprivate |
Definition at line 300 of file Queue.h.
References groupA, groupB, and theLocalGroup.
Referenced by findOldest().
◆ remoteReader() [1/2]
|
overrideprotectedvirtual |
Implements Ipc::BaseMultiQueue.
◆ remoteReader() [2/2]
|
protectedinherited |
◆ remotesCount()
|
overrideprotectedvirtual |
Implements Ipc::BaseMultiQueue.
◆ remotesIdOffset()
|
overrideprotectedvirtual |
Implements Ipc::BaseMultiQueue.
◆ stat()
|
inherited |
Definition at line 586 of file Queue.h.
References Ipc::BaseMultiQueue::inQueue(), Ipc::BaseMultiQueue::localReader(), Ipc::BaseMultiQueue::outQueue(), Ipc::BaseMultiQueue::remotesCount(), Ipc::BaseMultiQueue::remotesIdOffset(), and Ipc::BaseMultiQueue::theLocalProcessId.
◆ validProcessId()
Definition at line 253 of file Queue.cc.
Referenced by findOldest().
Member Data Documentation
◆ metadata
|
private |
Definition at line 303 of file Queue.h.
Referenced by FewToFewBiQueue().
◆ queues
|
private |
Definition at line 304 of file Queue.h.
Referenced by FewToFewBiQueue().
◆ readers
|
private |
Definition at line 305 of file Queue.h.
Referenced by FewToFewBiQueue().
◆ theLastPopProcessId
|
privateinherited |
Definition at line 231 of file Queue.h.
Referenced by Ipc::BaseMultiQueue::peek(), and Ipc::BaseMultiQueue::pop().
◆ theLocalGroup
|
private |
Definition at line 307 of file Queue.h.
Referenced by localGroup(), and remoteGroup().
◆ theLocalProcessId
|
protectedinherited |
Definition at line 228 of file Queue.h.
Referenced by findOldest(), Ipc::BaseMultiQueue::pop(), Ipc::BaseMultiQueue::push(), and Ipc::BaseMultiQueue::stat().
The documentation for this class was generated from the following files: