#include <splay.h>
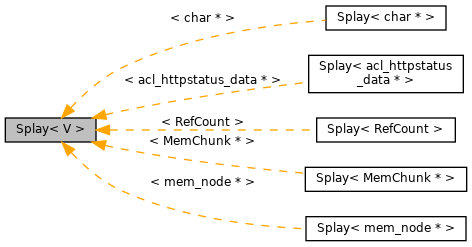
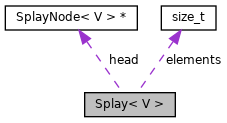
Public Types | |
typedef V | Value |
typedef int | SPLAYCMP(Value const &a, Value const &b) |
typedef void | SPLAYFREE(Value &) |
typedef SplayIterator< V > | iterator |
Public Member Functions | |
Splay () | |
template<class FindValue > | |
const Value * | find (FindValue const &, int(*compare)(FindValue const &a, Value const &b)) const |
const Value * | insert (const Value &, SPLAYCMP *) |
void | remove (Value const &, SPLAYCMP *compare) |
void | destroy (SPLAYFREE *=DefaultFree) |
const SplayNode< V > * | start () const |
const SplayNode< V > * | finish () const |
size_t | size () const |
bool | empty () const |
const_iterator | begin () const |
const_iterator | end () const |
template<typename ValueVisitor > | |
void | visit (ValueVisitor &) const |
left-to-right visit of all stored Values More... | |
template<class Visitor > | |
void | visitEach (Visitor &visitor) const |
template<class Visitor > | |
void | visit (Visitor &visitor) const |
Static Public Member Functions | |
static void | DefaultFree (Value &v) |
Public Attributes | |
const typedef SplayConstIterator< V > | const_iterator |
Private Member Functions | |
template<typename NodeVisitor > | |
void | visitEach (NodeVisitor &) const |
left-to-right walk through all nodes More... | |
Private Attributes | |
SplayNode< V > * | head |
size_t | elements |
Detailed Description
Member Typedef Documentation
◆ iterator
typedef SplayIterator<V> Splay< V >::iterator |
◆ SPLAYCMP
◆ SPLAYFREE
◆ Value
Constructor & Destructor Documentation
◆ Splay()
Member Function Documentation
◆ begin()
const SplayConstIterator< V > Splay< V >::begin |
Definition at line 388 of file splay.h.
Referenced by testHdrVisit().
◆ DefaultFree()
◆ destroy()
void Splay< V >::destroy | ( | SPLAYFREE * | free_func = DefaultFree | ) |
Definition at line 369 of file splay.h.
Referenced by mem_hdr::freeContent(), main(), testSplayOfNodes(), ACLDomainData::~ACLDomainData(), ACLHTTPStatus::~ACLHTTPStatus(), and ACLIP::~ACLIP().
◆ empty()
|
inline |
Definition at line 78 of file splay.h.
Referenced by ACLDomainData::empty(), ACLHTTPStatus::empty(), and ACLIP::empty().
◆ end()
const SplayConstIterator< V > Splay< V >::end |
Definition at line 395 of file splay.h.
Referenced by testHdrVisit().
◆ find()
const Splay< V >::Value * Splay< V >::find | ( | FindValue const & | value, |
int(*)(FindValue const &a, Value const &b) | compare | ||
) | const |
Definition at line 305 of file splay.h.
Referenced by MemPoolChunked::convertFreeCacheToChunkFreeCache(), mem_hdr::getBlockContainingLocation(), ACLServerNameData::match(), ACLDomainData::match(), ACLIP::match(), mem_hdr::nodeToRecieve(), testSplayOfNodes(), and mem_hdr::unionNotEmpty().
◆ finish()
Definition at line 359 of file splay.h.
Referenced by mem_hdr::dump(), mem_hdr::endOffset(), mem_hdr::freeDataUpto(), main(), and testSplayOfNodes().
◆ insert()
const Splay< V >::Value * Splay< V >::insert | ( | const Value & | value, |
SPLAYCMP * | compare | ||
) |
If the given value matches a stored one, returns that matching value. Otherwise, stores the given unique value and returns nil.
Definition at line 320 of file splay.h.
Referenced by mem_hdr::appendNode(), main(), MemChunk::MemChunk(), Acl::SplayInserter< DataValue >::Merge(), and testSplayOfNodes().
◆ remove()
Definition at line 336 of file splay.h.
Referenced by Acl::SplayInserter< DataValue >::Merge(), mem_hdr::unlink(), and MemChunk::~MemChunk().
◆ size()
Definition at line 381 of file splay.h.
Referenced by mem_hdr::copy(), Splay< char * >::empty(), main(), mem_hdr::nodeToRecieve(), and mem_hdr::size().
◆ start()
Definition at line 349 of file splay.h.
Referenced by mem_hdr::dump(), mem_hdr::freeDataUpto(), mem_hdr::lowestOffset(), main(), mem_hdr::nodeToRecieve(), and testSplayOfNodes().
◆ visit() [1/2]
Referenced by mem_hdr::debugDump(), ACLDomainData::dump(), ACLHTTPStatus::dump(), and ACLIP::dump().
◆ visit() [2/2]
◆ visitEach() [1/2]
|
private |
◆ visitEach() [2/2]
Member Data Documentation
◆ const_iterator
const typedef SplayConstIterator<V> Splay< V >::const_iterator |
◆ elements
◆ head
The documentation for this class was generated from the following file:
- include/splay.h
Introduction
- About Squid
- Why Squid?
- Squid Developers
- How to Donate
- How to Help Out
- Getting Squid
- Squid Source Packages
- Squid Deployment Case-Studies
- Squid Software Foundation
Documentation
- Quick Setup
- Configuration:
- FAQ and Wiki
- Guide Books:
- Non-English
- More...
Support
- Security Advisories
- Bugzilla Database
- Mailing lists
- Contacting us
- Commercial services
- Project Sponsors
- Squid-based products